java实现httpclient请求,并且请求中会传输MP4文件,也会传递其他参数,要求设置头部Content-Type: application/mp4
时间: 2024-06-08 15:09:12 浏览: 131
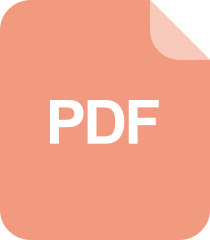
java使用httpclient发送post请求示例

可以使用Apache HttpClient库来实现HTTP请求,以下是一个示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.EntityBuilder;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
public class HttpClientExample {
public static void main(String[] args) throws ClientProtocolException, IOException {
String url = "http://example.com/upload"; // 上传接口地址
String filePath = "/path/to/file.mp4"; // MP4文件路径
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
// 设置请求头部
httpPost.setHeader("Content-Type", "application/mp4");
// 设置请求参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("name", "value"));
httpPost.setEntity(new UrlEncodedFormEntity(params));
// 设置上传文件
File file = new File(filePath);
InputStream inputStream = new FileInputStream(file);
HttpEntity fileEntity = EntityBuilder.create()
.setContentType(ContentType.APPLICATION_OCTET_STREAM)
.setBinary(inputStream)
.build();
// 组装请求实体,包括文件和参数
HttpEntity reqEntity = MultipartEntityBuilder.create()
.addBinaryBody("file", fileEntity, ContentType.APPLICATION_OCTET_STREAM, file.getName())
.addTextBody("name", "value")
.build();
httpPost.setEntity(reqEntity);
// 执行请求
HttpResponse httpResponse = client.execute(httpPost);
HttpEntity responseEntity = httpResponse.getEntity();
// 处理响应数据
System.out.println(responseEntity.getContent());
}
}
```
在这个示例中,我们使用`HttpPost`实例来构建一个POST请求,设置了请求头部`Content-Type: application/mp4`,并添加了一个MP4文件和其他参数到请求实体中。在执行请求后,我们可以从响应实体中获取响应数据。需要注意的是,这里使用了MultipartEntityBuilder来组装请求实体,因为请求中包含了文件和其他参数,而Multipart实体可以同时包含多个不同类型的HTTP实体。
阅读全文
相关推荐
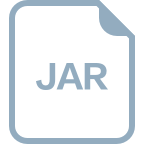
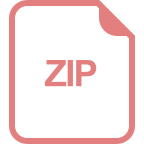
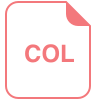
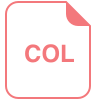
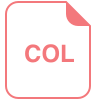
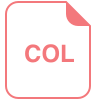
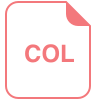
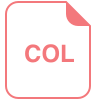
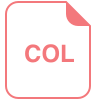
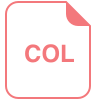
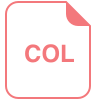
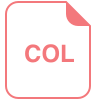
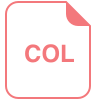
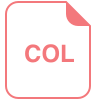
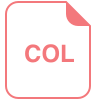
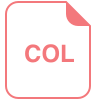
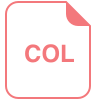