rules = [('西红柿','排骨'), ('排骨','西红柿'), ('袜子','鸡蛋'), ('茄子','排骨'), ('茄子','西红柿'), ('茄子','鸡蛋')] from mlxtend.frequent_patterns import apriori, association_rules # 计算频繁项集 freq_itemsets = apriori(df1, min_support=0.2, use_colnames=True) # 计算关联规则 rules = association_rules(freq_itemsets, metric="confidence", min_threshold=0.5) rules = rules.sort_values(by=['confidence'], ascending=False) pd.options.display.float_format = '{:.6f}'.format # 输出结果 # 支持度 support # 置信度 confidence print(rules[['antecedents', 'consequents', 'support', 'confidence']])。将这个代码,每一行都给出解释,说明为什么这么做代码,
时间: 2023-09-14 22:07:21 浏览: 83
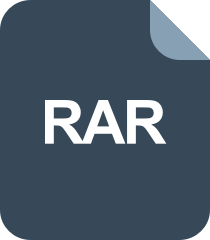
关联规则apriori算法
这段代码是用来计算关联规则的。具体来说,它使用了Apriori算法来计算频繁项集,并基于这些频繁项集计算关联规则。
代码中的第一行定义了一个包含商品之间关联关系的列表,每个元素都是一个二元组,表示两个商品之间有关联。这个列表被用于后续的计算。
第二行导入了`apriori`和`association_rules`函数,这两个函数都来自于`mlxtend.frequent_patterns`模块。`apriori`函数用于计算频繁项集,`association_rules`函数用于根据频繁项集计算关联规则。
第三行调用了`apriori`函数来计算频繁项集。`df1`是一个数据框,包含了所有的交易记录,`min_support=0.2`表示只考虑支持度不小于0.2的项集,`use_colnames=True`表示使用商品名称而不是编号来表示项集。
第四行调用了`association_rules`函数来计算关联规则。`freq_itemsets`是由`apriori`函数计算得到的频繁项集,`metric="confidence"`表示关联规则的质量指标是置信度,`min_threshold=0.5`表示只考虑置信度不小于0.5的规则。
第五行对关联规则按照置信度降序排列。
第六行设置了输出格式,保留小数点后6位。
第七行输出关联规则,包括前件、后件、支持度和置信度。
阅读全文
相关推荐
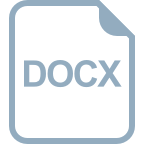


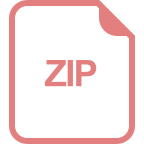
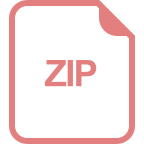
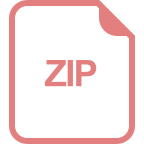
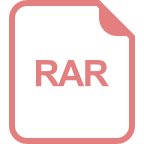
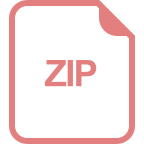
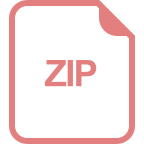
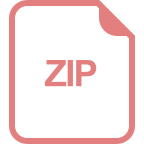
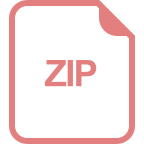
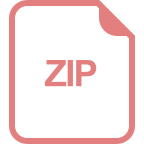
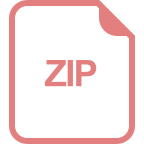