.net core将xml的请求实例转换为application/x-www-form-urlencoded模型
时间: 2023-07-29 19:13:36 浏览: 64
要将XML请求转换为application/x-www-form-urlencoded模型,您需要对XML请求进行解析,并将其转换为.NET对象。然后,您可以使用.NET的内置方法将.NET对象转换为application/x-www-form-urlencoded格式的字符串。
以下是一个示例,演示如何使用XmlSerializer将XML请求转换为.NET对象,并使用System.Net.Http.Formatting库将.NET对象转换为application/x-www-form-urlencoded格式的字符串:
```csharp
using System.Net.Http.Formatting;
using System.Xml.Serialization;
// 定义XML请求实体类
[XmlRoot("OTA_InventoryCheckRQ")]
public class InventoryCheckRequest
{
[XmlAttribute("EchoToken")]
public string EchoToken { get; set; }
[XmlAttribute("Password")]
public string Password { get; set; }
[XmlAttribute("PrimaryLangID")]
public string PrimaryLangID { get; set; }
[XmlAttribute("TimeStamp")]
public string TimeStamp { get; set; }
[XmlAttribute("UserName")]
public string UserName { get; set; }
[XmlAttribute("Version")]
public string Version { get; set; }
[XmlElement("HotelReservations")]
public HotelReservations HotelReservations { get; set; }
}
public class HotelReservations
{
[XmlElement("HotelReservation")]
public HotelReservation HotelReservation { get; set; }
}
public class HotelReservation
{
[XmlElement("RoomStay")]
public RoomStay RoomStay { get; set; }
[XmlElement("ResGlobalInfo")]
public ResGlobalInfo ResGlobalInfo { get; set; }
}
public class RoomStay
{
[XmlElement("RoomTypes")]
public RoomTypes RoomTypes { get; set; }
[XmlElement("RatePlans")]
public RatePlans RatePlans { get; set; }
[XmlElement("GuestCounts")]
public GuestCounts GuestCounts { get; set; }
[XmlElement("BasicPropertyInfo")]
public BasicPropertyInfo BasicPropertyInfo { get; set; }
}
public class RoomTypes
{
[XmlElement("RoomType")]
public RoomType RoomType { get; set; }
}
public class RoomType
{
[XmlAttribute("RoomTypeCode")]
public string RoomTypeCode { get; set; }
}
public class RatePlans
{
[XmlElement("RatePlan")]
public RatePlan RatePlan { get; set; }
}
public class RatePlan
{
[XmlAttribute("RatePlanCode")]
public string RatePlanCode { get; set; }
}
public class GuestCounts
{
[XmlElement("GuestCount")]
public List<GuestCount> GuestCount { get; set; }
}
public class GuestCount
{
[XmlAttribute("AgeQualifyingCode")]
public string AgeQualifyingCode { get; set; }
[XmlAttribute("Count")]
public int Count { get; set; }
}
public class BasicPropertyInfo
{
[XmlAttribute("HotelCode")]
public string HotelCode { get; set; }
}
public class ResGlobalInfo
{
[XmlElement("RoomCount")]
public int RoomCount { get; set; }
[XmlElement("MemberLevel")]
public string MemberLevel { get; set; }
[XmlElement("TimeSpan")]
public TimeSpan TimeSpan { get; set; }
}
public class TimeSpan
{
[XmlAttribute("End")]
public string End { get; set; }
[XmlAttribute("Start")]
public string Start { get; set; }
}
// 在控制器中处理请求
public async Task<IActionResult> ProcessRequest([FromBody] string xmlRequest)
{
// 解析XML请求
XmlSerializer serializer = new XmlSerializer(typeof(InventoryCheckRequest));
InventoryCheckRequest request;
using (StringReader reader = new StringReader(xmlRequest))
{
request = (InventoryCheckRequest)serializer.Deserialize(reader);
}
// 将.NET对象转换为application/x-www-form-urlencoded格式的字符串
var formData = new FormUrlEncodedContent(new Dictionary<string, string>
{
{ "EchoToken", request.EchoToken },
{ "Password", request.Password },
{ "PrimaryLangID", request.PrimaryLangID },
{ "TimeStamp", request.TimeStamp },
{ "UserName", request.UserName },
{ "Version", request.Version },
{ "HotelReservations.HotelReservation.RoomStay.RoomTypes.RoomType.RoomTypeCode", request.HotelReservations.HotelReservation.RoomStay.RoomTypes.RoomType.RoomTypeCode },
{ "HotelReservations.HotelReservation.RoomStay.RatePlans.RatePlan.RatePlanCode", request.HotelReservations.HotelReservation.RoomStay.RatePlans.RatePlan.RatePlanCode },
{ "HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[0].AgeQualifyingCode", request.HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[0].AgeQualifyingCode },
{ "HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[0].Count", request.HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[0].Count.ToString() },
{ "HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[1].AgeQualifyingCode", request.HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[1].AgeQualifyingCode },
{ "HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[1].Count", request.HotelReservations.HotelReservation.RoomStay.GuestCounts.GuestCount[1].Count.ToString() },
{ "HotelReservations.HotelReservation.RoomStay.BasicPropertyInfo.HotelCode", request.HotelReservations.HotelReservation.RoomStay.BasicPropertyInfo.HotelCode },
{ "HotelReservations.HotelReservation.ResGlobalInfo.RoomCount", request.HotelReservations.HotelReservation.ResGlobalInfo.RoomCount.ToString() },
{ "HotelReservations.HotelReservation.ResGlobalInfo.MemberLevel", request.HotelReservations.HotelReservation.ResGlobalInfo.MemberLevel },
{ "HotelReservations.HotelReservation.ResGlobalInfo.TimeSpan.Start", request.HotelReservations.HotelReservation.ResGlobalInfo.TimeSpan.Start },
{ "HotelReservations.HotelReservation.ResGlobalInfo.TimeSpan.End", request.HotelReservations.HotelReservation.ResGlobalInfo.TimeSpan.End }
});
// 发送POST请求
HttpClient client = new HttpClient();
HttpResponseMessage response = await client.PostAsync("https://example.com", formData);
// 处理响应并返回结果
string responseBody = await response.Content.ReadAsStringAsync();
return Ok(responseBody);
}
```
请注意,上述代码仅供参考。您需要根据自己的实际情况进行修改和调整。
相关推荐
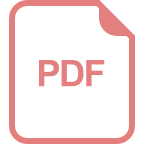
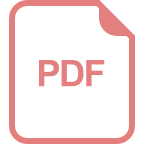
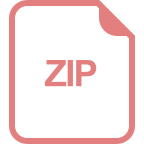














