怎么将字符串转换成application/x-www-form-urlencoded
时间: 2023-09-09 12:05:07 浏览: 330
### 回答1:
可以使用urlencode函数将字符串转换成application/x-www-form-urlencoded格式。例如,在C语言中,可以使用以下代码实现:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char str[] = "hello world";
char *encoded_str = NULL;
encoded_str = (char *)malloc(strlen(str) * 3 + 1);
if (encoded_str == NULL) {
printf("Memory allocation failed!\n");
return -1;
}
int i, j = ;
for (i = ; i < strlen(str); i++) {
if ((str[i] >= 'A' && str[i] <= 'Z') ||
(str[i] >= 'a' && str[i] <= 'z') ||
(str[i] >= '' && str[i] <= '9') ||
(str[i] == '-' || str[i] == '_' || str[i] == '.' || str[i] == '~')) {
encoded_str[j++] = str[i];
} else {
sprintf(encoded_str + j, "%%%02X", str[i]);
j += 3;
}
}
encoded_str[j] = '\';
printf("Original string: %s\n", str);
printf("Encoded string: %s\n", encoded_str);
free(encoded_str);
return ;
}
这段代码将字符串"hello world"转换成了"hello%20world"的形式。
### 回答2:
将字符串转换为`application/x-www-form-urlencoded`格式,可以使用URL编码的方式进行转换。
URL编码是一种将字符串中的特殊字符转换为%xx格式的编码方法,其中xx表示特殊字符在ASCII表中的十六进制表示。在`application/x-www-form-urlencoded`格式中,所有的特殊字符都会被编码。
下面是一个使用Java实现的示例代码:
```java
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
public class UrlEncodeExample {
public static void main(String[] args) {
String str = "Hello World!";
try {
String encodedStr = URLEncoder.encode(str, "UTF-8");
System.out.println(encodedStr);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
```
在上述示例中,我们使用了`URLEncoder`类的`encode`方法将字符串`"Hello World!"`进行编码。编码后的字符串为`Hello+World%21`。编码时指定了字符集为`UTF-8`。
在`application/x-www-form-urlencoded`格式中,空格将会被转换为`+`号,而特殊字符(如`!`)将会被转换为%xx格式。
你可以根据自己的需求修改上述代码,并将其集成到你的项目中。
### 回答3:
将字符串转换为application/x-www-form-urlencoded格式的过程如下:
1. 首先,将字符串按照要求的格式进行编码。这包括将字符串中的特殊字符进行转义,例如将空格编码为%20,将逗号编码为%2C,将斜杠编码为%2F等。
2. 然后,将编码后的字符串中的每个字符都转换为字节序列,并使用ASCII码进行表示。
3. 接着,将每个字节序列转换为十六进制表示,并在前面添加%符号。
4. 最后,将所有转换后的字节序列按照顺序连接起来,并以&符号作为分隔符。
举例说明:假设有一个字符串"hello world"需要转换为application/x-www-form-urlencoded格式。按照上述步骤进行转换:
1. 编码字符串,将空格转义为%20: "hello%20world"。
2. 将编码后的字符串转换为字节序列,并使用ASCII码表示: "h" 为 104, "e"为101, "l"为108, "o"为111,空格为32,以此类推。
3. 将每个字节序列转换为十六进制表示,并添加%符号: 104转换为%68,101转换为%65,108转换为%6C,111转换为%6F,32转换为%20。
4. 将所有转换后的字节序列连接起来,并以&符号作为分隔符: %68%65%6C%6C%6F%20%77%6F%72%6C%64。
最终得到的字符串就是将原始字符串转换为application/x-www-form-urlencoded格式的结果。
阅读全文
相关推荐
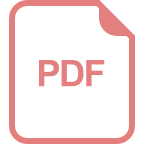
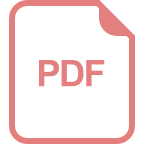
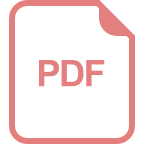















