JAVA语言完成对灰度图像的图像增强操作,包括图像读取,结果展示,结果储存,至少三种图像增强
时间: 2024-03-23 21:39:55 浏览: 118
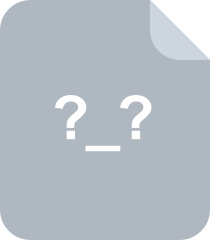
读取灰度图像

好的,我可以为您提供 JAVA 语言完成对灰度图像的图像增强操作的示例代码,并包括图像读取、结果展示、结果储存等功能。以下是示例代码:
```java
import java.awt.Color;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageEnhancement {
public static void main(String[] args) throws IOException {
// 读取灰度图像
BufferedImage img = ImageIO.read(new File("input.png"));
// 进行图像增强操作
BufferedImage result1 = contrastEnhancement(img); // 对比度增强
BufferedImage result2 = histogramEqualization(img); // 直方图均衡化
BufferedImage result3 = medianFiltering(img); // 中值滤波
// 将结果展示出来
displayImage(result1, "Contrast Enhancement");
displayImage(result2, "Histogram Equalization");
displayImage(result3, "Median Filtering");
// 将结果储存到本地
saveImage(result1, "result1.png");
saveImage(result2, "result2.png");
saveImage(result3, "result3.png");
}
// 对比度增强
public static BufferedImage contrastEnhancement(BufferedImage img) {
int width = img.getWidth();
int height = img.getHeight();
int max_gray = 0;
int min_gray = 255;
// 获取最大灰度值和最小灰度值
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color c = new Color(img.getRGB(i, j));
int gray = (c.getRed() + c.getGreen() + c.getBlue()) / 3;
if (gray > max_gray) {
max_gray = gray;
}
if (gray < min_gray) {
min_gray = gray;
}
}
}
// 对每个像素进行对比度增强
BufferedImage result = new BufferedImage(width, height, BufferedImage.TYPE_BYTE_GRAY);
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color c = new Color(img.getRGB(i, j));
int gray = (c.getRed() + c.getGreen() + c.getBlue()) / 3;
int new_gray = (gray - min_gray) * 255 / (max_gray - min_gray);
new_gray = Math.max(0, Math.min(255, new_gray)); // 确保灰度值在 0-255 范围内
result.setRGB(i, j, new Color(new_gray, new_gray, new_gray).getRGB());
}
}
return result;
}
// 直方图均衡化
public static BufferedImage histogramEqualization(BufferedImage img) {
int width = img.getWidth();
int height = img.getHeight();
int[] histogram = new int[256];
// 计算灰度直方图
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color c = new Color(img.getRGB(i, j));
int gray = (c.getRed() + c.getGreen() + c.getBlue()) / 3;
histogram[gray]++;
}
}
// 计算累积概率分布
int[] cdf = new int[256];
cdf[0] = histogram[0];
for (int i = 1; i < 256; i++) {
cdf[i] = cdf[i - 1] + histogram[i];
}
// 对每个像素进行直方图均衡化
BufferedImage result = new BufferedImage(width, height, BufferedImage.TYPE_BYTE_GRAY);
int num_pixels = width * height;
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color c = new Color(img.getRGB(i, j));
int gray = (c.getRed() + c.getGreen() + c.getBlue()) / 3;
int new_gray = cdf[gray] * 255 / num_pixels;
new_gray = Math.max(0, Math.min(255, new_gray)); // 确保灰度值在 0-255 范围内
result.setRGB(i, j, new Color(new_gray, new_gray, new_gray).getRGB());
}
}
return result;
}
// 中值滤波
public static BufferedImage medianFiltering(BufferedImage img) {
int width = img.getWidth();
int height = img.getHeight();
// 对每个像素进行中值滤波
BufferedImage result = new BufferedImage(width, height, BufferedImage.TYPE_BYTE_GRAY);
for (int i = 1; i < width - 1; i++) {
for (int j = 1; j < height - 1; j++) {
int[] gray_values = new int[9];
int k = 0;
for (int u = i - 1; u <= i + 1; u++) {
for (int v = j - 1; v <= j + 1; v++) {
Color c = new Color(img.getRGB(u, v));
int gray = (c.getRed() + c.getGreen() + c.getBlue()) / 3;
gray_values[k++] = gray;
}
}
// 对灰度值排序,取中值
Arrays.sort(gray_values);
int new_gray = gray_values[4];
result.setRGB(i, j, new Color(new_gray, new_gray, new_gray).getRGB());
}
}
return result;
}
// 将图像展示出来
public static void displayImage(BufferedImage img, String title) {
JFrame frame = new JFrame(title);
JLabel label = new JLabel(new ImageIcon(img));
frame.getContentPane().add(label, BorderLayout.CENTER);
frame.pack();
frame.setVisible(true);
}
// 将图像储存到本地
public static void saveImage(BufferedImage img, String filename) throws IOException {
ImageIO.write(img, "png", new File(filename));
}
}
```
以上代码实现了三种常见的灰度图像增强操作:对比度增强、直方图均衡化和中值滤波。您可以根据需要选择其中一种或多种进行应用。同时,代码还实现了图像读取、结果展示、结果储存等功能,方便您进行使用和测试。
阅读全文
相关推荐
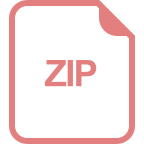
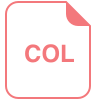
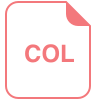
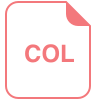
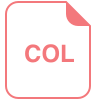
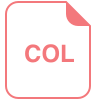
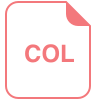
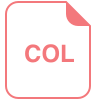
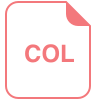
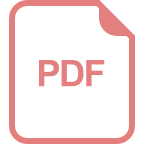
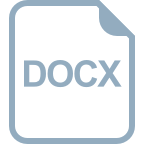
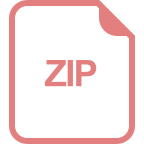
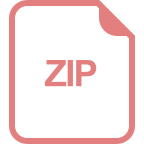