QPlainTextEdit自定义拖动框选事件
时间: 2024-06-08 11:05:40 浏览: 8
QPlainTextEdit并没有提供拖动框选事件的接口,但我们可以通过继承QPlainTextEdit并重载其鼠标事件来实现自定义拖动框选事件。
具体实现步骤如下:
1. 重载QPlainTextEdit的mousePressEvent、mouseMoveEvent、mouseReleaseEvent三个鼠标事件。
2. 在mousePressEvent中记录下鼠标按下时的位置,同时调用基类的mousePressEvent。
3. 在mouseMoveEvent中判断当前鼠标是否处于按下状态,如果是,则计算出当前鼠标位置与鼠标按下时的位置之间的矩形区域,并调用QPlainTextEdit的setExtraSelections方法来设置这个矩形区域的选中状态。
4. 在mouseReleaseEvent中清空之前设置的额外选中区域,并调用基类的mouseReleaseEvent。
代码示例:
```python
from PyQt5.QtWidgets import QPlainTextEdit
from PyQt5.QtCore import Qt, QRect
from PyQt5.QtGui import QTextCursor, QPalette, QTextOption, QColor, QTextCharFormat
class DragSelectPlainTextEdit(QPlainTextEdit):
def __init__(self, parent=None):
super().__init__(parent)
self._dragging = False
self._start_pos = None
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self._dragging = True
self._start_pos = event.pos()
super().mousePressEvent(event)
def mouseMoveEvent(self, event):
if self._dragging:
end_pos = event.pos()
selection_rect = QRect(self._start_pos, end_pos).normalized()
self.setExtraSelections([QTextCursor(self.document()).selection()])
self.setExtraSelections([QTextCursor(self.document()).selection(), QTextEdit.ExtraSelection()])
self.extraSelections()[1].format.setBackground(QColor("#C0C0C0"))
self.extraSelections()[1].cursor = QTextCursor(self.document())
self.extraSelections()[1].cursor.setPosition(self.cursorForPosition(selection_rect.topLeft()).position())
self.extraSelections()[1].cursor.setPosition(self.cursorForPosition(selection_rect.bottomRight()).position(), QTextCursor.KeepAnchor)
super().mouseMoveEvent(event)
def mouseReleaseEvent(self, event):
if self._dragging:
self.setExtraSelections([QTextCursor(self.document()).selection()])
self._dragging = False
super().mouseReleaseEvent(event)
```
在上述代码中,我们通过QTextEdit.ExtraSelection()创建了一个额外的选中区域,然后设置其背景色并将其与文本光标一起设置到QPlainTextEdit中,从而实现了自定义的拖动框选事件。
相关推荐
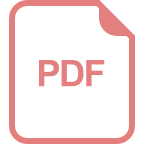
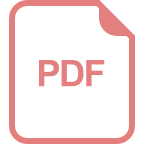














