QPlainTextEdit怎样自定义框选文字然后拖拽
时间: 2023-11-27 10:52:01 浏览: 30
可以通过重载 QPlainTextEdit 的 mousePressEvent、mouseMoveEvent、mouseReleaseEvent 三个事件来实现自定义框选文字然后拖拽的功能。
具体实现步骤如下:
1. 在 mousePressEvent 中记录下起始位置,清空选中的文本。
2. 在 mouseMoveEvent 中计算出当前鼠标所在位置,然后设置选中的文本。
3. 在 mouseReleaseEvent 中根据选中的文本来确定是否要进行拖拽操作。
下面是一个简单的示例代码,演示了如何自定义框选文字并进行拖拽:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QPlainTextEdit
from PyQt5.QtCore import Qt, QPoint
class MyTextEdit(QPlainTextEdit):
def __init__(self, parent=None):
super(MyTextEdit, self).__init__(parent)
self._is_dragging = False
self._start_pos = QPoint(0, 0)
self._end_pos = QPoint(0, 0)
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self._start_pos = event.pos()
self._end_pos = event.pos()
self.setTextCursor(self.cursorForPosition(event.pos()))
self.setTextCursor(self.textCursor())
self.setTextCursor(Qt.IBeamCursor)
self.clearFocus()
def mouseMoveEvent(self, event):
if event.buttons() == Qt.LeftButton:
self._end_pos = event.pos()
start = self.cursorForPosition(self._start_pos)
end = self.cursorForPosition(self._end_pos)
cursor = self.textCursor()
cursor.setPosition(start.position(), QTextCursor.MoveAnchor)
cursor.setPosition(end.position(), QTextCursor.KeepAnchor)
self.setTextCursor(cursor)
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
if self._is_dragging:
self._is_dragging = False
self.setCursor(Qt.IBeamCursor)
else:
self._is_dragging = True
def mouseDoubleClickEvent(self, event):
if event.button() == Qt.LeftButton:
self.clearFocus()
if __name__ == '__main__':
app = QApplication([])
window = QMainWindow()
text_edit = MyTextEdit()
window.setCentralWidget(text_edit)
window.show()
app.exec_()
```
在这个示例中,我们重载了 QPlainTextEdit 的 mousePressEvent、mouseMoveEvent、mouseReleaseEvent 和 mouseDoubleClickEvent 四个事件。其中,mousePressEvent 记录下了起始位置,并清空了选中的文本;mouseMoveEvent 计算出当前鼠标所在位置,并设置选中的文本;mouseReleaseEvent 根据选中的文本来确定是否要进行拖拽操作;mouseDoubleClickEvent 清除了焦点。
相关推荐
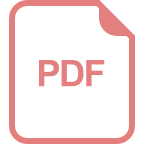














