java实现下载功能的代码
时间: 2023-10-12 19:05:10 浏览: 41
以下是 Java 实现文件下载的代码:
```java
import java.io.*;
import java.net.*;
public class FileDownloader {
public static void main(String[] args) {
String fileUrl = "http://example.com/file.pdf"; // 文件的 URL 地址
String saveDir = "/home/user/downloads/"; // 文件保存的目录
try {
URL url = new URL(fileUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
int responseCode = httpConn.getResponseCode();
// 检查服务器响应是否为 OK
if (responseCode == HttpURLConnection.HTTP_OK) {
// 获取文件名和文件大小
String fileName = "";
String disposition = httpConn.getHeaderField("Content-Disposition");
if (disposition != null) {
int startIndex = disposition.indexOf("filename=");
if (startIndex > 0) {
fileName = disposition.substring(startIndex + 10, disposition.length() - 1);
}
} else {
fileName = fileUrl.substring(fileUrl.lastIndexOf("/") + 1, fileUrl.length());
}
int fileSize = httpConn.getContentLength();
// 创建输入流和输出流
InputStream inputStream = httpConn.getInputStream();
FileOutputStream outputStream = new FileOutputStream(saveDir + fileName);
// 读取文件,并将文件保存到本地磁盘
byte[] buffer = new byte[4096];
int bytesRead = -1;
int totalBytesRead = 0;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
totalBytesRead += bytesRead;
}
outputStream.close();
inputStream.close();
System.out.println("File downloaded successfully!");
} else {
System.out.println("Error: Server returned response code " + responseCode);
}
httpConn.disconnect();
} catch (IOException ex) {
System.out.println("Error: " + ex.getMessage());
}
}
}
```
请注意,如果要下载的文件需要身份验证或需要代理服务器,则需要先设置合适的 HTTP 请求头部和代理服务器。在这个示例代码中没有包含这些情况的处理。
相关推荐
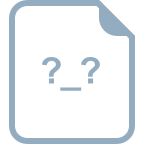
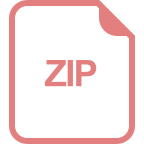
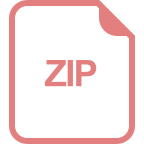
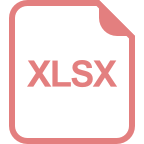
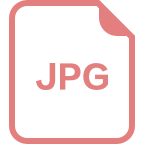
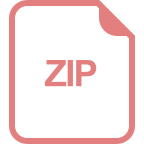
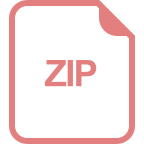