为这段代码增加详细注释import java.io.*; public class FileMerge { public static void main(String[] args) { if (args.length != 3) { System.out.println("Usage: java FileMerge source1 source2 target"); return; } String sourceFile1 = args[0]; String sourceFile2 = args[1]; String targetFile = args[2]; try (BufferedInputStream in1 = new BufferedInputStream(new FileInputStream(sourceFile1)); BufferedInputStream in2 = new BufferedInputStream(new FileInputStream(sourceFile2)); BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(targetFile))) { byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = in1.read(buffer)) != -1) { out.write(buffer, 0, bytesRead); } while ((bytesRead = in2.read(buffer)) != -1) { out.write(buffer, 0, bytesRead); } System.out.println("File merge completed."); } catch (FileNotFoundException e) { System.err.println("File not found: " + e.getMessage()); } catch (IOException e) { System.err.println("IOException: " + e.getMessage()); } } }
时间: 2024-02-14 17:09:36 浏览: 19
好的,以下是对该代码进行详细注释的版本:
```java
import java.io.*;
public class FileMerge {
public static void main(String[] args) {
// 判断命令行参数是否为3个,如果不是则输出用法并返回
if (args.length != 3) {
System.out.println("Usage: java FileMerge source1 source2 target");
return;
}
// 读取命令行参数中的源文件1、源文件2和目标文件名
String sourceFile1 = args[0];
String sourceFile2 = args[1];
String targetFile = args[2];
try (
// 创建输入流1,使用缓冲区读取文件内容
BufferedInputStream in1 = new BufferedInputStream(new FileInputStream(sourceFile1));
// 创建输入流2,使用缓冲区读取文件内容
BufferedInputStream in2 = new BufferedInputStream(new FileInputStream(sourceFile2));
// 创建输出流,使用缓冲区写入文件内容
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(targetFile))
) {
byte[] buffer = new byte[1024]; // 缓冲区大小为1024字节
int bytesRead; // 每次读取的字节数
// 从输入流1读取数据,写入输出流
while ((bytesRead = in1.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead); // 将读取到的字节数组写入输出流中
}
// 从输入流2读取数据,写入输出流
while ((bytesRead = in2.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead); // 将读取到的字节数组写入输出流中
}
System.out.println("File merge completed.");
} catch (FileNotFoundException e) { // 捕获文件未找到异常
System.err.println("File not found: " + e.getMessage());
} catch (IOException e) { // 捕获IO异常
System.err.println("IOException: " + e.getMessage());
}
}
}
```
注释中添加了对每个方法和变量的详细说明,以及对异常的捕获和处理进行了注释。
相关推荐
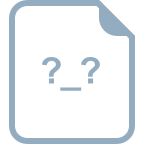
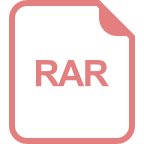
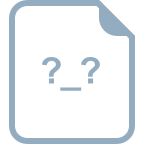















