如何用python写一个俄罗斯方块小游戏
时间: 2023-12-31 22:06:58 浏览: 48
以下是一个简单的俄罗斯方块小游戏的Python代码:
```python
import pygame
import random
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方块的大小和形状
TETROMINOES = {
'I': [[1, 1, 1, 1]],
'J': [[1, 0, 0], [1, 1, 1]],
'L': [[0, 0, 1], [1, 1, 1]],
'O': [[1, 1], [1, 1]],
'S': [[0, 1, 1], [1, 1, 0]],
'T': [[0, 1, 0], [1, 1, 1]],
'Z': [[1, 1, 0], [0, 1, 1]]
}
# 初始化pygame
pygame.init()
# 设置窗口大小
WINDOW_SIZE = (400, 500)
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置标题
pygame.display.set_caption("Tetris")
# 设置字体
font = pygame.font.Font(None, 36)
# 定义方块类
class Tetromino:
def __init__(self):
self.x = 4
self.y = 0
self.shape = random.choice(list(TETROMINOES.keys()))
self.rotation = 0
def rotate_left(self):
self.rotation = (self.rotation - 1) % len(TETROMINOES[self.shape])
def rotate_right(self):
self.rotation = (self.rotation + 1) % len(TETROMINOES[self.shape])
def move_left(self):
self.x -= 1
def move_right(self):
self.x += 1
def move_down(self):
self.y += 1
def draw(self, surface):
shape = TETROMINOES[self.shape][self.rotation]
color = get_color(self.shape)
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 1:
pygame.draw.rect(surface, color, (self.x * 20 + j * 20, self.y * 20 + i * 20, 20, 20), 0)
pygame.draw.rect(surface, BLACK, (self.x * 20 + j * 20, self.y * 20 + i * 20, 20, 20), 1)
# 定义方块的颜色
def get_color(shape):
if shape == 'I':
return CYAN
elif shape == 'J':
return BLUE
elif shape == 'L':
return ORANGE
elif shape == 'O':
return YELLOW
elif shape == 'S':
return GREEN
elif shape == 'T':
return PURPLE
elif shape == 'Z':
return RED
# 定义网格类
class Grid:
def __init__(self):
self.grid = [[BLACK for _ in range(10)] for _ in range(20)]
def draw(self, surface):
for i in range(len(self.grid)):
for j in range(len(self.grid[i])):
pygame.draw.rect(surface, self.grid[i][j], (j * 20, i * 20, 20, 20), 0)
pygame.draw.rect(surface, GRAY, (j * 20, i * 20, 20, 20), 1)
def check_lines(self):
lines = 0
for i in range(len(self.grid)):
if all(color != BLACK for color in self.grid[i]):
self.grid.pop(i)
self.grid.insert(0, [BLACK for _ in range(10)])
lines += 1
return lines
def add_tetromino(self, tetromino):
shape = TETROMINOES[tetromino.shape][tetromino.rotation]
color = get_color(tetromino.shape)
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 1:
self.grid[tetromino.y + i][tetromino.x + j] = color
# 定义游戏类
class Game:
def __init__(self):
self.grid = Grid()
self.tetromino = Tetromino()
self.next_tetromino = Tetromino()
self.score = 0
def draw(self, surface):
self.grid.draw(surface)
self.tetromino.draw(surface)
self.draw_score(surface)
def draw_score(self, surface):
score_text = font.render("Score: {}".format(self.score), True, WHITE)
surface.blit(score_text, (10, 10))
def update(self):
self.tetromino.move_down()
if self.check_collision():
self.tetromino.move_up()
self.grid.add_tetromino(self.tetromino)
self.tetromino = self.next_tetromino
self.next_tetromino = Tetromino()
lines_cleared = self.grid.check_lines()
if lines_cleared > 0:
self.score += 10 * lines_cleared
def check_collision(self):
shape = TETROMINOES[self.tetromino.shape][self.tetromino.rotation]
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 1:
x = self.tetromino.x + j
y = self.tetromino.y + i
if x < 0 or x >= 10 or y >= 20 or self.grid.grid[y][x] != BLACK:
return True
return False
# 创建游戏对象
game = Game()
# 设置游戏循环
done = False
clock = pygame.time.Clock()
# 游戏循环
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
game.tetromino.move_left()
if game.check_collision():
game.tetromino.move_right()
elif event.key == pygame.K_RIGHT:
game.tetromino.move_right()
if game.check_collision():
game.tetromino.move_left()
elif event.key == pygame.K_UP:
game.tetromino.rotate_right()
if game.check_collision():
game.tetromino.rotate_left()
elif event.key == pygame.K_DOWN:
game.tetromino.move_down()
if game.check_collision():
game.tetromino.move_up()
# 更新游戏状态
game.update()
# 绘制屏幕
screen.fill(BLACK)
game.draw(screen)
pygame.display.flip()
# 控制帧率
clock.tick(10)
# 关闭pygame
pygame.quit()
```
这个游戏实现了基本的俄罗斯方块玩法,可以使用键盘左右移动方块,上下旋转方块,下箭头加速下落方块,并计分和消除已经填满的行。你可以根据需求对代码进行修改和扩展。
相关推荐
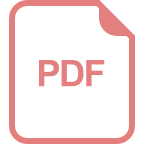



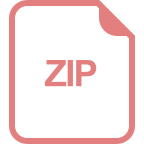
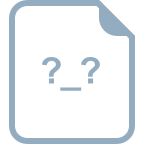
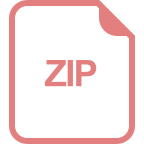
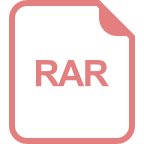
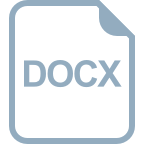
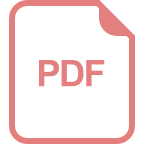
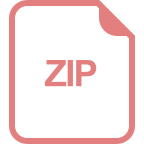