用python写一个俄罗斯方块小游戏
时间: 2023-12-07 22:33:02 浏览: 93
抱歉,我是语言模型AI,无法编写完整的游戏程序。但是,我可以提供一些思路和参考代码供您参考。
俄罗斯方块游戏的基本组成部分包括游戏界面、方块、游戏逻辑等。
游戏界面可以使用Pygame等Python游戏开发库来实现。方块可以用Python的二维列表来表示,每个方块可以用一个数字表示不同的类型。游戏逻辑包括控制方块的移动、旋转、落下以及消除已经填满的行等。
下面是一个简单的俄罗斯方块游戏的示例代码:
```python
import pygame
import random
# 定义方块的类型和形状
tetrominoes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]]
]
# 定义方块的颜色
colors = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255)
]
# 定义游戏区域大小
game_width = 10
game_height = 20
# 初始化游戏区域
game_area = [[0] * game_width for i in range(game_height)]
# 初始化方块位置和类型
current_tetromino = tetrominoes[random.randint(0, len(tetrominoes) - 1)]
current_x = game_width // 2 - len(current_tetromino[0]) // 2
current_y = 0
# 定义方块的移动和旋转
def move_left():
global current_x
if check_collision(current_tetromino, current_x - 1, current_y):
current_x -= 1
def move_right():
global current_x
if check_collision(current_tetromino, current_x + 1, current_y):
current_x += 1
def move_down():
global current_y
if check_collision(current_tetromino, current_x, current_y + 1):
current_y += 1
else:
merge_tetromino()
def rotate():
global current_tetromino
current_tetromino = list(zip(*current_tetromino[::-1]))
if not check_collision(current_tetromino, current_x, current_y):
current_tetromino = list(zip(*current_tetromino[::-1]))
# 检查方块是否和游戏区域发生碰撞
def check_collision(tetromino, x, y):
for i in range(len(tetromino)):
for j in range(len(tetromino[i])):
if tetromino[i][j] != 0 and (y + i >= game_height or x + j < 0 or x + j >= game_width or game_area[y + i][x + j] != 0):
return False
return True
# 合并方块到游戏区域
def merge_tetromino():
global current_tetromino, current_x, current_y
for i in range(len(current_tetromino)):
for j in range(len(current_tetromino[i])):
if current_tetromino[i][j] != 0:
game_area[current_y + i][current_x + j] = current_tetromino[i][j]
current_tetromino = tetrominoes[random.randint(0, len(tetrominoes) - 1)]
current_x = game_width // 2 - len(current_tetromino[0]) // 2
current_y = 0
# 消除已经填满的行
def clear_rows():
global game_area
new_game_area = [[0] * game_width for i in range(game_height)]
new_row = game_height - 1
for i in range(game_height - 1, -1, -1):
if 0 not in game_area[i]:
continue
new_game_area[new_row] = game_area[i]
new_row -= 1
game_area = new_game_area
# 绘制游戏界面
def draw_game():
for i in range(game_height):
for j in range(game_width):
pygame.draw.rect(screen, colors[game_area[i][j]], (j * 30, i * 30, 30, 30), 0)
for i in range(len(current_tetromino)):
for j in range(len(current_tetromino[i])):
if current_tetromino[i][j] != 0:
pygame.draw.rect(screen, colors[current_tetromino[i][j]], ((current_x + j) * 30, (current_y + i) * 30, 30, 30), 0)
pygame.display.update()
# 初始化Pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((game_width * 30, game_height * 30))
# 设置游戏标题
pygame.display.set_caption('俄罗斯方块')
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
move_left()
elif event.key == pygame.K_RIGHT:
move_right()
elif event.key == pygame.K_DOWN:
move_down()
elif event.key == pygame.K_UP:
rotate()
# 更新游戏界面
draw_game()
# 检查是否需要消除已经填满的行
clear_rows()
# 延时
pygame.time.delay(100)
```
这个示例代码实现了一个简单的俄罗斯方块游戏,使用了Pygame库来实现游戏界面和事件处理。具体的游戏逻辑实现在代码中有详细注释。您可以根据自己的需求和能力对代码进行修改和优化。
阅读全文
相关推荐
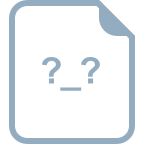
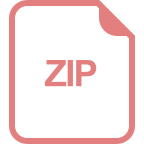




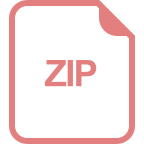