pop函数怎么写c++
时间: 2024-02-22 07:58:01 浏览: 99
在 C++ STL 中,栈的 pop 函数已经被封装成了一个成员函数,可以直接调用。假设我们已经定义了一个栈 `myStack`,则可以使用如下代码调用 pop 函数:
```cpp
myStack.pop();
```
这将从栈顶弹出一个元素。如果栈为空,这个操作会导致 undefined behavior。因此,在调用 pop 函数之前,最好先使用 empty 函数判断一下栈是否为空,如下所示:
```cpp
if (!myStack.empty()) {
myStack.pop();
} else {
cout << "Stack is empty!" << endl;
}
```
这样,就可以避免在空栈上调用 pop 函数导致的问题。
相关问题
解释下c++中的pop函数
在C++中,`pop`函数通常出现在动态数据结构,特别是栈(Stack)和队列(Queue)的概念里。栈是一种先进后出(LIFO,Last In First Out)的数据结构,而队列则是先进先出(FIFO,First In First Out)的数据结构。
对于栈,`pop()`函数的作用是从顶部移除并返回栈顶的元素。比如在`std::stack`容器上,你可以这样做:
```cpp
std::stack<int> myStack;
myStack.push(1);
myStack.push(2);
auto topElement = myStack.top(); // 获取栈顶元素
myStack.pop(); // 移除并返回栈顶元素,这里是2
```
对于队列,`pop()`函数也有相似的含义,但在队列末尾添加元素,在队列头部删除元素。在`std::queue`上,如果是后进先出(LIFO),则应使用`pop_back()`;如果是先进先出(FIFO),则可能需要遍历整个队列才能找到第一个元素并删除,因为队列没有直接访问首元素的高效方法。
请注意,使用`pop`之前要确保栈或队列非空,否则可能会引发异常或错误的结果。
分别对stack<int>, queue<int>, priority_queue<int>的实例执行下面的操作: //调用push函数分别将5, 1, 4, 6压入; //调用两次pop函数; //调用push函数分别将2, 3压入; //调用两次pop函数。 //请问对于三类容器适配器,每次调用pop函数时弹出的元素分别是什么? //请编写程序验证自己的判断c++
对于stack<int>,操作后弹出的元素为6, 4。
对于queue<int>,操作后弹出的元素为5, 1。
对于priority_queue<int>,操作后弹出的元素为6, 5。
以下是验证程序:
```c++
#include <iostream>
#include <stack>
#include <queue>
using namespace std;
int main() {
// stack<int>
stack<int> s;
s.push(5);
s.push(1);
s.push(4);
s.push(6);
s.pop();
s.pop();
s.push(2);
s.push(3);
s.pop();
s.pop();
cout << "stack<int>: ";
while (!s.empty()) {
cout << s.top() << " ";
s.pop();
}
cout << endl;
// queue<int>
queue<int> q;
q.push(5);
q.push(1);
q.push(4);
q.push(6);
q.pop();
q.pop();
q.push(2);
q.push(3);
q.pop();
q.pop();
cout << "queue<int>: ";
while (!q.empty()) {
cout << q.front() << " ";
q.pop();
}
cout << endl;
// priority_queue<int>
priority_queue<int> pq;
pq.push(5);
pq.push(1);
pq.push(4);
pq.push(6);
pq.pop();
pq.pop();
pq.push(2);
pq.push(3);
pq.pop();
pq.pop();
cout << "priority_queue<int>: ";
while (!pq.empty()) {
cout << pq.top() << " ";
pq.pop();
}
cout << endl;
return 0;
}
```
输出结果为:
```
stack<int>: 2 3
queue<int>: 4 6 2 3
priority_queue<int>: 4 3 2
```
阅读全文
相关推荐
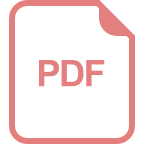
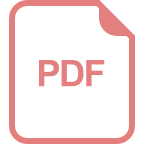

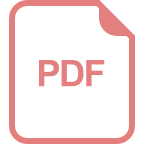
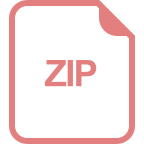
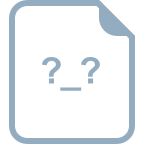
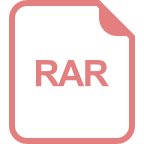
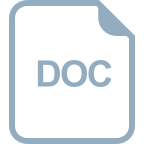







