帮我写一份winform进度条控件,进度条颜色可自定义,并且进度条带有按钮单击可以将视频播放到指定位置,进度条还得支持圆角属性
时间: 2023-05-16 15:04:49 浏览: 196
好的,我可以帮您编写一份winform进度条控件。以下是代码示例:
```
using System.Drawing;
using System.Windows.Forms;
public class CustomProgressBar : ProgressBar
{
private Color _barColor = Color.Green;
private bool _roundedCorners = false;
public Color BarColor
{
get { return _barColor; }
set { _barColor = value; }
}
public bool RoundedCorners
{
get { return _roundedCorners; }
set { _roundedCorners = value; }
}
protected override void OnPaint(PaintEventArgs e)
{
Rectangle rect = e.ClipRectangle;
if (_roundedCorners)
{
int radius = Height / 2;
GraphicsPath path = new GraphicsPath();
path.AddArc(rect.X, rect.Y, radius, radius, 180, 90);
path.AddArc(rect.X + rect.Width - radius, rect.Y, radius, radius, 270, 90);
path.AddArc(rect.X + rect.Width - radius, rect.Y + rect.Height - radius, radius, radius, 0, 90);
path.AddArc(rect.X, rect.Y + rect.Height - radius, radius, radius, 90, 90);
path.CloseFigure();
e.Graphics.FillPath(new SolidBrush(BackColor), path);
}
else
{
e.Graphics.FillRectangle(new SolidBrush(BackColor), rect);
}
rect.Width = (int)(rect.Width * ((double)Value / Maximum)) - 4;
rect.Height -= 4;
if (rect.Width > 0)
{
if (_roundedCorners)
{
int radius = rect.Height / 2;
GraphicsPath path = new GraphicsPath();
path.AddArc(rect.X, rect.Y, radius, radius, 180, 90);
path.AddArc(rect.X + rect.Width - radius, rect.Y, radius, radius, 270, 90);
path.AddLine(rect.X + rect.Width, rect.Y + radius, rect.X + rect.Width, rect.Y + rect.Height - radius);
path.AddArc(rect.X + rect.Width - radius, rect.Y + rect.Height - radius, radius, radius, 0, 90);
path.AddArc(rect.X, rect.Y + rect.Height - radius, radius, radius, 90, 90);
path.CloseFigure();
e.Graphics.FillPath(new SolidBrush(_barColor), path);
}
else
{
e.Graphics.FillRectangle(new SolidBrush(_barColor), rect);
}
}
}
}
```
使用方法:
1. 将以上代码复制到您的项目中。
2. 在您的窗体中添加一个ProgressBar控件。
3. 在窗体的Load事件中,将ProgressBar控件替换为CustomProgressBar控件。
示例代码:
```
private void Form1_Load(object sender, EventArgs e)
{
CustomProgressBar progressBar = new CustomProgressBar();
progressBar.Location = new Point(10, 10);
progressBar.Size = new Size(200, 30);
progressBar.Minimum = 0;
progressBar.Maximum = 100;
progressBar.Value = 50;
progressBar.BarColor = Color.Blue;
progressBar.RoundedCorners = true;
this.Controls.Add(progressBar);
}
```
这样就可以在窗体中显示一个自定义的进度条控件了。进度条的颜色可以通过BarColor属性进行设置,圆角属性可以通过RoundedCorners属性进行设置。按钮单击可以将视频播放到指定位置的功能需要您自己实现。
阅读全文
相关推荐
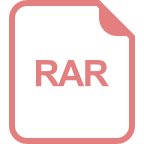


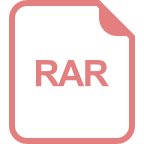
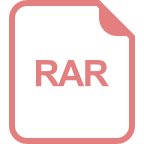
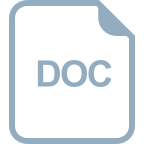
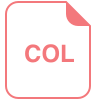



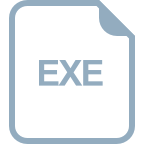
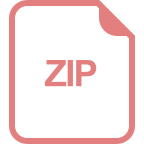