帮我写一个ros2 下 点云订阅/ss话题,与rpc通信的代码,要求用C++编程,整洁高效
时间: 2024-03-14 22:44:36 浏览: 187
好的,下面是一个示例代码,用于在ROS2下订阅PointCloud2类型的消息,并通过rpc通信进行传输。
```cpp
#include <iostream>
#include <memory>
#include "rclcpp/rclcpp.hpp"
#include "sensor_msgs/msg/point_cloud2.hpp"
#include "rpc.hpp"
using namespace std::chrono_literals;
class PointCloudSubscriber : public rclcpp::Node
{
public:
PointCloudSubscriber() : Node("point_cloud_subscriber")
{
// 创建订阅者,订阅PointCloud2类型的消息
subscriber_ = this->create_subscription<sensor_msgs::msg::PointCloud2>(
"/ss", 10, std::bind(&PointCloudSubscriber::callback, this, std::placeholders::_1));
// 初始化RPC客户端
rpc_client_ = std::make_shared<rpc::client>("127.0.0.1", 9000);
}
private:
void callback(const sensor_msgs::msg::PointCloud2::SharedPtr msg)
{
// 处理消息
std::cout << "Received point cloud message with " << msg->width * msg->height << " points." << std::endl;
// 将点云数据转换为字符串,进行RPC通信传输
std::ostringstream oss;
oss << *msg;
std::string point_cloud_str = oss.str();
// 调用RPC服务端的接口
std::string result = rpc_client_->call("process_point_cloud", point_cloud_str).as<std::string>();
// 处理RPC服务端返回的结果
std::cout << "RPC call returned: " << result << std::endl;
}
rclcpp::Subscription<sensor_msgs::msg::PointCloud2>::SharedPtr subscriber_;
std::shared_ptr<rpc::client> rpc_client_;
};
int main(int argc, char* argv[])
{
rclcpp::init(argc, argv);
rclcpp::spin(std::make_shared<PointCloudSubscriber>());
rclcpp::shutdown();
return 0;
}
```
在上面的代码中,我们定义了一个名为`PointCloudSubscriber`的类,该类继承自`rclcpp::Node`。在类的构造函数中,我们创建了一个订阅者,用于订阅`/ss`话题上的PointCloud2类型的消息。同时,我们也初始化了一个RPC客户端,用于与RPC服务端进行通信。
在订阅到消息后,我们首先将点云数据转换为字符串,并通过RPC客户端调用RPC服务端的接口。RPC服务端会接收到点云数据,并进行处理,最后将处理结果返回给RPC客户端。在客户端收到RPC服务端返回的结果后,我们可以对结果进行处理。
需要注意的是,在使用RPC通信传输数据时,需要将数据转换为字符串,以便在不同的机器间进行传输和解析。在本示例中,我们使用`std::ostringstream`将点云数据转换为字符串。在RPC服务端接收到数据后,需要将字符串转换回点云数据。
阅读全文
相关推荐




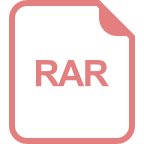
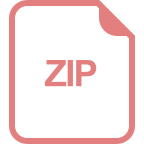
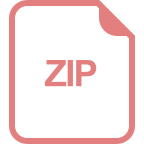
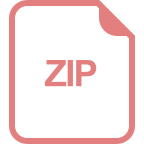
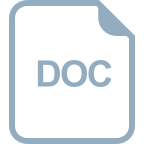
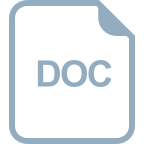
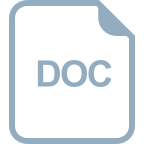
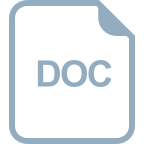
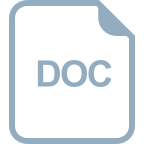

