CoAP协议是什么,报文格式,如何解析?
时间: 2023-02-07 16:40:00 浏览: 544
CoAP协议(Constrained Application Protocol)是一种基于UDP的网络协议,主要用于资源受限的设备(如物联网设备)之间的通信。CoAP报文由三部分组成:首部、有效载荷和选项。
首部由四个字节组成,包含版本号、类型、代码、报文标识符和设备状态码等信息。
有效载荷是可选的,包含报文的具体内容。
选项是可选的,用于提供其他元数据信息。
要解析CoAP报文,需要按照上述格式进行解析。首先,从报文开头读取首部,然后根据首部中的信息来解析有效载荷和选项。对于有效载荷和选项,需要根据相应的格式进行解析,以便获取所需的信息。
阅读全文
相关推荐
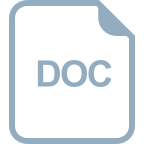
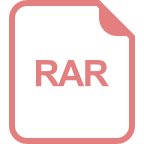
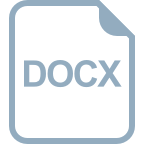
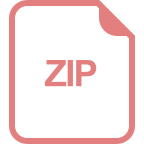
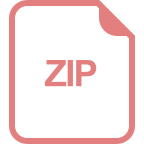
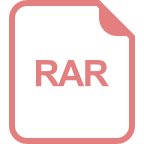
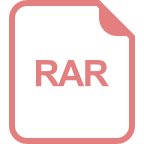
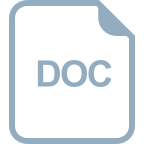
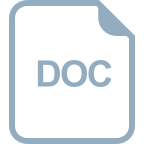
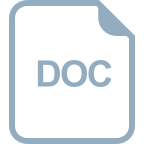
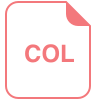
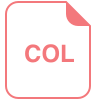
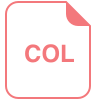
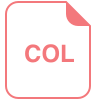
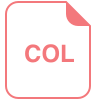
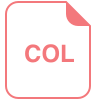
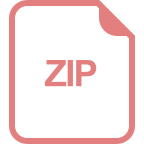