python函数实现图片滤波后的均方误差
时间: 2024-04-08 18:33:29 浏览: 80
要计算图片滤波后的均方误差,你可以按照以下步骤实现一个Python函数:
1. 导入必要的库和模块,如NumPy和OpenCV:
```python
import numpy as np
import cv2
```
2. 创建一个函数来计算均方误差(Mean Squared Error,MSE):
```python
def calculate_mse(image1, image2):
squared_diff = np.square(image1 - image2)
mse = np.mean(squared_diff)
return mse
```
3. 读取原始图像和滤波后的图像:
```python
original_image = cv2.imread('path_to_original_image.jpg', 0) # 以灰度图像方式读取原始图像
filtered_image = cv2.imread('path_to_filtered_image.jpg', 0) # 以灰度图像方式读取滤波后的图像
```
4. 调用函数计算均方误差:
```python
mse = calculate_mse(original_image, filtered_image)
print("Mean Squared Error:", mse)
```
请确保将 `'path_to_original_image.jpg'` 和 `'path_to_filtered_image.jpg'` 替换为你自己的图像文件路径。
这个函数将返回滤波后图像与原始图像之间的均方误差值。
阅读全文
相关推荐
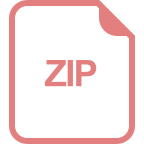
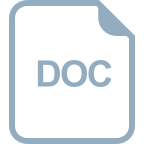

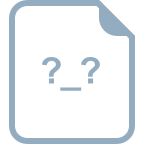
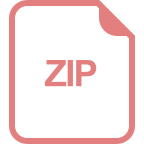
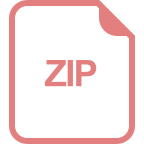
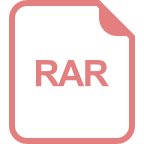
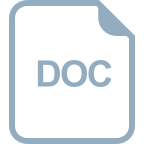
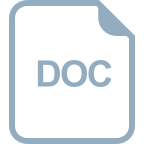
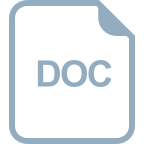
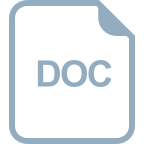
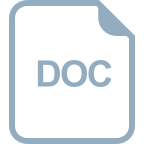
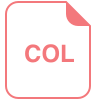
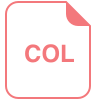


