设计一个用于用户登陆的网页,要求: 首页:输入用户名和密码,提交给第一个Servlet。 Servlet1:如果用户名和密码分别是“admin”和”123456”,则进入登陆成功页面。否则跳转到登陆失败页面。 登陆失败页面:页面上有一个超链接回到首页让用户重新输入。 登陆成功页面:一个欢迎页面,显示登陆的用户名。用户名下方有一个超链接,链接到第二个Servlet。 Servlet2:在页面上显示当前时间。
时间: 2024-01-24 20:17:44 浏览: 68
以下是一个简单的用户登陆网页的设计:
首页(index.jsp):
```
<!DOCTYPE html>
<html>
<head>
<title>用户登陆</title>
</head>
<body>
<h1>用户登陆</h1>
<form action="login" method="post">
<label>用户名:</label>
<input type="text" name="username"><br>
<label>密码:</label>
<input type="password" name="password"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
当用户在首页输入用户名和密码并提交后,会将输入的数据发送到名为“login”的Servlet中。
Servlet1(LoginServlet.java):
```
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public LoginServlet() {
super();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if (username.equals("admin") && password.equals("123456")) {
request.getSession().setAttribute("username", username);
response.sendRedirect("welcome");
} else {
response.sendRedirect("loginFailed");
}
}
}
```
这个Servlet会接收到来自首页的POST请求,并检查输入的用户名和密码是否正确。如果正确,则将用户名保存到Session中,并重定向到欢迎页面;否则重定向到登陆失败页面。
登陆失败页面(loginFailed.jsp):
```
<!DOCTYPE html>
<html>
<head>
<title>登陆失败</title>
</head>
<body>
<h1>登陆失败</h1>
<p>用户名或密码错误,请重新输入。</p>
<a href="index.jsp">返回首页</a>
</body>
</html>
```
如果输入的用户名和密码不正确,则会重定向到这个页面。
欢迎页面(welcome.jsp):
```
<!DOCTYPE html>
<html>
<head>
<title>欢迎</title>
</head>
<body>
<h1>欢迎</h1>
<p>欢迎您,<%= session.getAttribute("username") %>。</p>
<a href="showTime">显示当前时间</a>
</body>
</html>
```
这个页面会显示登陆成功后的欢迎信息,并提供一个超链接,链接到第二个Servlet。
Servlet2(ShowTimeServlet.java):
```
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/showTime")
public class ShowTimeServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public ShowTimeServlet() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日 HH:mm:ss");
String now = sdf.format(new Date());
response.getWriter().write("当前时间是:" + now);
}
}
```
这个Servlet会接收到来自欢迎页面的GET请求,并生成当前时间的字符串,然后将其输出到页面上。
以上就是一个简单的用户登陆网页的设计。
相关推荐
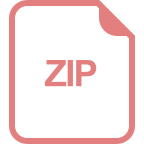
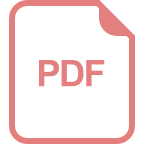
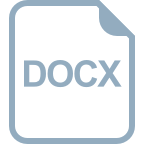
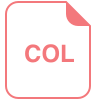
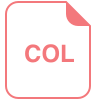
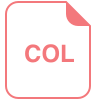
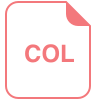
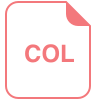









