WPF使用Image实现给图片换颜色
时间: 2024-09-27 22:05:13 浏览: 54
在Windows Presentation Foundation (WPF) 中,如果你想要改变Image加载的图片的颜色,你可以利用`System.Windows.Media.Imaging`库中的`BitmapImage`类和`DrawingVisual`、`DrawingContext`来进行图像处理。以下是一个简单的示例,展示了如何创建一个新的颜色转换后的`BitmapImage`:
```csharp
using System;
using System.IO;
using System.Windows;
using System.Windows.Media;
using System.Windows.Media.Imaging;
public class ColoredImageConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, string language)
{
BitmapImage originalImage = (BitmapImage)value;
// 创建一个新的位图上下文
DrawingContext context = null;
using (var visual = new DrawingVisual())
{
context = visual.RenderOpen();
// 设置画布大小和原始图片大小一致
var drawSize = new Size(originalImage.PixelWidth, originalImage.PixelHeight);
visual.Viewport = new Rect(0, 0, drawSize.Width, drawSize.Height);
// 创建渐变色刷并应用到Image上
var colorGradient = new LinearGradientBrush(
new Point(0, 0),
new Point(0, 1),
Brushes.Blue, // 原始颜色
Brushes.Yellow, // 新颜色
new Point(0.5, 0.5));
// 使用画刷绘制图像
context.DrawImage(originalImage, new Rect(0, 0, drawSize.Width, drawSize.Height), colorGradient);
}
// 返回新的彩色BitmapImage
return new BitmapImage(new MemoryStream(context.Pixels));
}
public object ConvertBack(object value, Type targetType, object parameter, string language)
{
throw new NotImplementedException();
}
}
// 在XAML中使用这个值转换器
<Image x:Name="MyImage" Source="{Binding MyOriginalImageSource, Converter={StaticResource ColorConverter}}">
<Image.Style>
<Style TargetType="Image">
<Setter Property="Background" Value="Transparent" />
</Style>
</Image.Style>
</Image>
// 其中ColorConverter就是上述定义的ColoredImageConverter实例,并将其添加到资源字典中
<local:ColoredImageConverter x:Key="ColorConverter" />
```
在这个例子中,我们将图片从蓝色转换为黄色。你可以根据需要修改渐变的颜色和位置。同时,请确保替换`MyOriginalImageSource`为你实际使用的图片源。
阅读全文
相关推荐
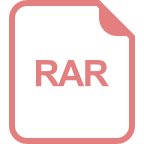
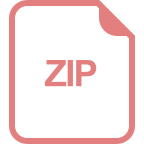
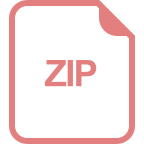















