WPF的Image实现给图片换颜色
时间: 2024-09-27 08:05:19 浏览: 51
在WPF(Windows Presentation Foundation)中,你可以使用`System.Windows.Media.Imaging`命名空间下的`BitmapImage`或`WriteableBitmap`类来加载图片,并对图像进行颜色变换。以下是一个简单的例子,展示了如何使用`ColorMatrix`和`DrawingVisual`来改变图片的颜色:
```csharp
using System;
using System.IO;
using System.Windows;
using System.Windows.Media;
using System.Windows.Media.Imaging;
public class ColorChanger
{
public static void ChangeImageColor(string imagePath, SolidColorBrush color)
{
// 加载图片
BitmapImage image = new BitmapImage(new Uri(imagePath));
// 创建一个可写的位图,以便进行修改
WriteableBitmap writableBitmap = new WriteableBitmap(image.Width, image.Height);
writableBitmap.Lock();
// 使用画刷创建颜色矩阵
ColorMatrix colorMatrix = new ColorMatrix(new float[,]
{
color.R / 255f, 0, 0, 0, 0,
0, color.G / 255f, 0, 0, 0,
0, 0, color.B / 255f, 0, 0,
0, 0, 0, 1, 0
});
// 创建绘图视觉并应用颜色矩阵
DrawingVisual drawingVisual = new DrawingVisual();
using (var context = drawingVisual.RenderOpen())
{
var drawContext = context.CreateDrawingContext();
drawContext.DrawImage(writableBitmap, new Rect(0, 0, writableBitmap.PixelWidth, writableBitmap.PixelHeight));
drawContext.TransformMatrix = Matrix.Identity;
// 应用颜色矩阵到图像
var transform = VisualTreeHelper.DumpVisualTree(drawingVisual).Descendants().First(d => d is ImageSource) as ImageSource;
if (transform != null)
{
var matrixTransform = new MatrixTransform(colorMatrix);
transform.RenderTransform = matrixTransform;
}
}
// 将绘制后的图像复制回写入的位图
drawingVisual.Render(writableBitmap);
// 解锁并保存更改
writableBitmap.Unlock();
// 设置新的源
image.Source = writableBitmap;
}
}
// 调用示例
string imagePath = "path_to_your_image.jpg";
SolidColorBrush color = new SolidColorBrush(Colors.Blue); // 替换成你需要的颜色
ColorChanger.ChangeImageColor(imagePath, color);
```
这个代码首先加载图片,然后创建一个可写的位图,接着定义一个颜色矩阵来表示你要应用的新颜色。然后,在一个新的`DrawingVisual`中,我们将原图应用了颜色变换,最后更新了位图图像的源。
阅读全文
相关推荐
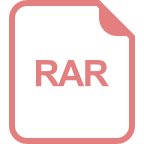
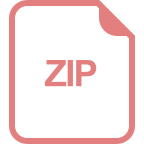
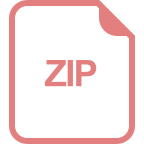



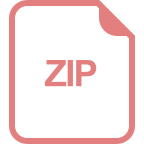
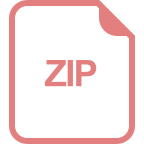
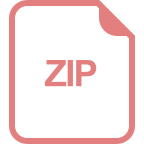
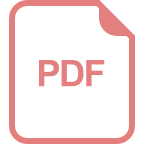
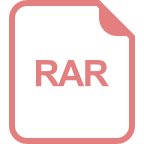
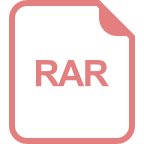
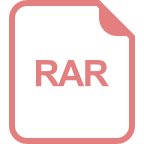
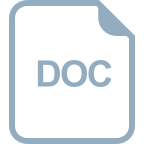
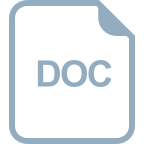
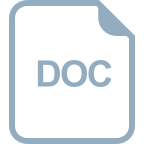
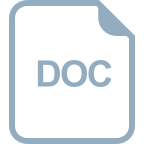

