用vue3实现登录注册界面和书籍管理首页,写出vue界面案例代码
时间: 2024-09-23 14:07:24 浏览: 41
在Vue3中,创建登录注册界面和书籍管理首页的基本结构通常包括组件化设计,这里我会提供一些基本的模板代码示例。首先,我们将创建两个独立的组件:Login.vue 和 BookList.vue。
**1. Login.vue (登录注册组件)**
```html
<template>
<div class="login-container">
<h2>登录/注册</h2>
<form @submit.prevent="handleSubmit">
<input v-model="username" placeholder="用户名" type="text">
<input v-model="password" placeholder="密码" type="password">
<button type="submit">登录 / 注册</button>
</form>
</div>
</template>
<script setup>
import { ref } from 'vue';
const username = ref('');
const password = ref('');
async function handleSubmit() {
// 这里可以发送异步请求到服务器验证用户信息
}
</script>
<style scoped>
.login-container {
/* 样式省略 */
}
</style>
```
**2. BookList.vue (书籍管理首页组件)**
```html
<template>
<div class="book-list-container">
<h2>书籍管理</h2>
<ul>
<!-- 使用v-for遍历数据 -->
<li v-for="(book, index) in books" :key="index">
{{ book.title }} - {{ book.author }}
</li>
</ul>
<button @click="addBook">添加新书</button>
</div>
</template>
<script setup>
// 假设我们有一个store来管理书籍数据
import { ref, watchEffect } from 'vue';
import { useStore } from './store';
const store = useStore();
const books = ref(store.state.books);
function addBook() {
// 添加书籍到store中
store.commit('ADD_BOOK', {
title: 'New Book',
author: 'Unknown'
});
}
watchEffect(() => {
// 当books状态改变时更新UI
books.value.forEach((book) => console.log(book));
});
</script>
<style scoped>
.book-list-container {
/* 样式省略 */
}
</style>
```
这两个组件需要配合Vue Router、Vuex等库进行路由切换和状态管理。记得安装并引入对应的依赖,并在主App文件中导入和使用这两个组件以及相应的路由器配置。
阅读全文
相关推荐
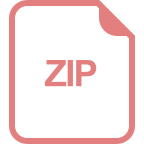
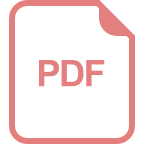
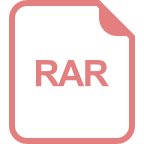
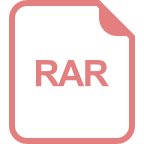
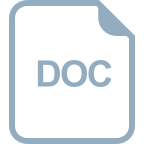
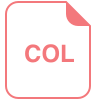
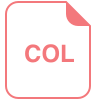
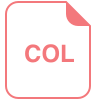
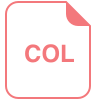
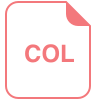
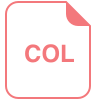
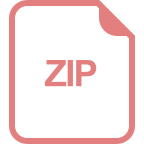
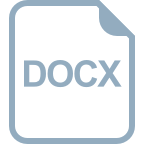