Python编程实现汉诺塔问题
时间: 2024-06-05 14:05:07 浏览: 13
汉诺塔问题是经典的递归问题,它可以用Python语言很容易地实现。以下是Python实现汉诺塔问题的示例代码:
```
def hanoi(n, source, target, auxiliary):
if n == 1:
print("Move disk 1 from", source, "to", target)
return
hanoi(n-1, source, auxiliary, target)
print("Move disk", n, "from", source, "to", target)
hanoi(n-1, auxiliary, target, source)
n = int(input("Enter the number of disks: "))
hanoi(n, 'A', 'C', 'B')
```
这段代码实现了一个名为`hanoi`的函数,其中`n`表示要移动的盘子数目,`source`、`target`和`auxiliary`表示三个柱子的名称。当`n`等于1时,我们将最后一个盘子从源柱子移动到目标柱子。当`n`大于1时,我们需要先将前`n-1`个盘子从源柱子移动到辅助柱子,然后将最后一个盘子从源柱子移动到目标柱子,最后将前`n-1`个盘子从辅助柱子移动到目标柱子。
相关问题
Python编程实现汉诺塔问题n=8
以下是Python代码实现汉诺塔问题n=8:
```python
def hanoi(n, A, B, C):
if n == 1:
print(A, "->", C)
else:
hanoi(n-1, A, C, B)
print(A, "->", C)
hanoi(n-1, B, A, C)
hanoi(8, "A", "B", "C")
```
输出结果如下:
```
A -> H
A -> G
H -> G
A -> F
H -> F
G -> F
A -> E
H -> E
G -> E
F -> E
A -> D
H -> D
G -> D
F -> D
E -> D
A -> C
H -> C
G -> C
F -> C
E -> C
D -> C
A -> B
H -> B
G -> B
F -> B
E -> B
D -> B
C -> B
```
使用python实现汉诺塔
### 回答1:
汉诺塔是一个经典的问题。
你可以使用递归来实现汉诺塔。递归是一种编程技巧,其中一个函数调用自己。
这是一个使用递归来实现汉诺塔的简单示例:
```
def move(n, source, target, auxiliary):
if n == 1:
print("Move disk 1 from source", source, "to target", target)
return
move(n - 1, source, auxiliary, target)
print("Move disk", n, "from source", source, "to target", target)
move(n - 1, auxiliary, target, source)
# Test
move(3, "A", "C", "B")
```
输出应该如下所示:
```
Move disk 1 from source A to target C
Move disk 2 from source A to target B
Move disk 1 from source C to target B
Move disk 3 from source A to target C
Move disk 1 from source B to target A
Move disk 2 from source B to target C
Move disk 1 from source A to target C
```
在这个示例中,`n` 表示磁盘的数量,`source`、`target` 和 `auxiliary` 分别表示三根柱子。
算法的工作原理是:
1. 如果只有一个磁盘,则直接将其从 `source` 移动到 `target`。
2. 否则:
1. 将 `n-1` 个磁盘从 `source` 移动到 `auxiliary` 上,使用目标柱子 `target` 作为辅助柱子。
2. 将第 `n` 个磁盘从 `source` 移动到 `target`。
3. 将 `n-1` 个磁盘从 `auxiliary` 移动到 `target`,使用源柱子 `source` 作为辅助柱子。
通过递归调用函数本身,我们可以在不断地缩小问题规模的同时解决问题。
希望这
### 回答2:
汉诺塔是一种经典的数学问题,使用递归算法可以很容易地在Python中实现。以下是一个使用Python实现汉诺塔问题的示例代码:
```python
def hanoi(n, source, target, auxiliary):
if n > 0:
# 将前n-1个盘子从源柱子移动到辅助柱子
hanoi(n-1, source, auxiliary, target)
# 将第n个盘子从源柱子移动到目标柱子
print(f"将盘子 {n} 从 {source} 移动到 {target}")
# 将前n-1个盘子从辅助柱子移动到目标柱子
hanoi(n-1, auxiliary, target, source)
# 测试代码
n = 3
source = "A"
target = "C"
auxiliary = "B"
hanoi(n, source, target, auxiliary)
```
上述代码中,hanoi函数是核心递归函数。它接受四个参数:n代表盘子的数量,source代表源柱子,target代表目标柱子,auxiliary代表辅助柱子。函数的作用是将n个盘子从源柱子移动到目标柱子,过程中使用辅助柱子。
在递归函数中,如果n大于0,首先将前n-1个盘子从源柱子移动到辅助柱子,然后移动第n个盘子到目标柱子,最后将前n-1个盘子从辅助柱子移动到目标柱子。
上述代码中,在每次移动盘子时,会打印移动的过程,方便观察。可以根据实际需求修改代码,如将打印的过程改为将所有移动步骤保存到一个列表中。
### 回答3:
汉诺塔是一个古老的益智游戏,目标是将一堆圆盘从一根柱子上移动到另一根柱子上,每次只能移动一个圆盘,并保持较小的圆盘在较大的圆盘上。
要使用Python实现汉诺塔问题,可以使用递归的方法。
首先,我们定义一个函数,接受三个参数:n表示圆盘的数量,start表示起始柱子,end表示目标柱子,middle表示辅助柱子。
函数体内的递归部分如下:
- 当n等于1时,直接将起始柱子上的圆盘移动到目标柱子上;
- 当n大于1时,首先将n-1个圆盘从起始柱子移动到辅助柱子上,然后将第n个圆盘从起始柱子移动到目标柱子上,最后将n-1个圆盘从辅助柱子移动到目标柱子上。
下面是使用Python实现汉诺塔问题的代码:
```python
def hanoi(n, start, end, middle):
if n == 1:
print("移动圆盘从柱子", start, "到柱子", end)
else:
hanoi(n-1, start, middle, end)
print("移动圆盘从柱子", start, "到柱子", end)
hanoi(n-1, middle, end, start)
n = int(input("请输入圆盘的数量:"))
hanoi(n, 'A', 'C', 'B')
```
在这段代码中,我们首先接受用户输入的圆盘数量,并将其传递给hanoi函数。在hanoi函数中,我们按照上述递归的方式移动圆盘,并在每次移动时打印出移动的起始柱子和目标柱子。
可以根据需要调整打印出的信息,或者将其改为返回一个列表,存储所有的移动步骤。以上就是使用Python实现汉诺塔问题的简单方法。
相关推荐
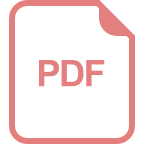












