使用c语言创建消息队列
时间: 2023-09-02 08:14:22 浏览: 124
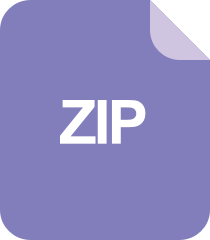
6Cpp,c语言消息队列源码,c语言项目
在C语言中,可以使用系统提供的消息队列函数集来创建消息队列。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 1024
struct message {
long msg_type;
char msg_data[MAX_MSG_SIZE];
};
int main(int argc, char* argv[]) {
int msgid;
key_t key;
struct message msg;
// 生成一个唯一的key
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(EXIT_FAILURE);
}
// 创建消息队列
if ((msgid = msgget(key, 0666 | IPC_CREAT)) == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送消息
msg.msg_type = 1;
strcpy(msg.msg_data, "Hello, world!");
if (msgsnd(msgid, &msg, sizeof(msg), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg), 0, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", msg.msg_data);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
在上述代码中,首先使用 `ftok` 函数生成一个唯一的key,然后使用 `msgget` 函数创建消息队列。发送消息使用 `msgsnd` 函数,接收消息使用 `msgrcv` 函数。最后使用 `msgctl` 函数删除消息队列。
在使用消息队列时,需要注意以下几个问题:
- 消息队列的key需要保证唯一性;
- 发送和接收的消息结构体需要一致;
- 发送和接收时需要指定消息类型;
- 消息队列的大小是有限制的,需要根据实际需求设置合适的大小;
- 需要注意消息队列的并发访问问题。
阅读全文
相关推荐
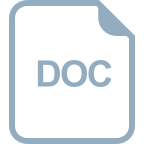
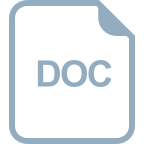
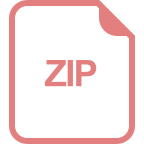
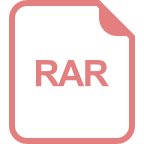
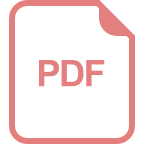
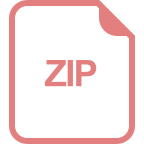
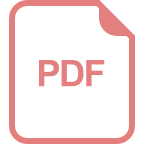
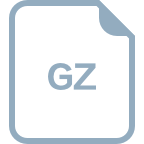
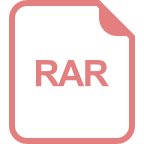
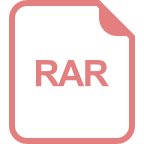
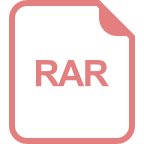
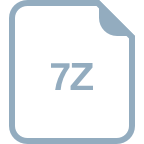
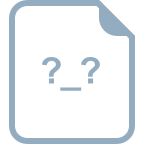
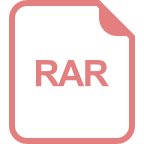
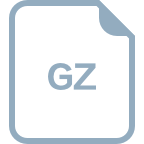
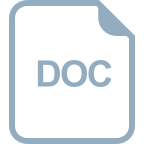
