HDLC verilog代码
时间: 2024-12-26 14:16:32 浏览: 9
### HDLC Protocol Implementation in Verilog
The High-Level Data Link Control (HDLC) protocol can be implemented using finite state machines (FSMs) and shift registers due to its requirement of handling data streams with specific framing rules. Below is an example of how such a protocol might be realized within Verilog.
#### Module Definition
A basic structure for implementing the HDLC receiver includes defining inputs like clock (`clk`), reset (`rst_n`), incoming serial data (`rx_data`), as well as outputs including received byte (`data_out`) and flag indicating completion or error conditions (`done`, `error`). Additionally, internal signals will manage states transitions and shifting operations.
```verilog
module hdlc_rx (
input wire clk,
input wire rst_n,
input wire rx_data,
output reg [7:0] data_out,
output reg done,
output reg error
);
```
#### Internal Signals Declaration
Internal wires and registers are declared to handle intermediate computations during each phase of reception:
- A register array holds bits being collected from the serial stream.
- Counters track progress through bytes.
- Flags indicate special cases encountered while processing frames.
```verilog
reg [7:0] shift_reg;
integer i;
wire start_flag = &{shift_reg[0], ~shift_reg[1]};
// ... other declarations ...
```
#### Finite State Machine States Enumeration
Define all possible operational stages involved when receiving packets according to HDLC specifications. This typically involves waiting for flags, collecting actual payload information between them, checking integrity via checksums/cyclic redundancy checks (CRC).
```verlog
typedef enum logic [2:0] {
IDLE,
RECEIVE_FLAG,
COLLECT_DATA,
CHECK_CRC,
ERROR_STATE
} state_t;
state_t current_state, next_state;
```
#### Combinational Logic For Next-State Determination
Based on present status along with new events detected over time steps defined by system clocks cycles, determine what should happen next regarding overall operation flow control inside this entity instance.
```verilog
always @(*) begin : proc_next_state
case(current_state)
IDLE: if(start_flag) next_state = RECEIVE_FLAG;
else next_state = IDLE;
RECEIVE_FLAG: /* similar pattern matching */
// ...
COLLECT_DATA: /* process incoming bits into buffer */
// ...
CHECK_CRC: /* validate frame correctness before signaling end-of-frame */
// ...
default: next_state = ERROR_STATE;
endcase
end
```
#### Sequential Logic To Update Current Status And Outputs
Use non-blocking assignments here so changes take effect only after completing evaluation across entire design hierarchy at every positive edge transition observed upon global timing reference signal provided externally.
```verilog
always @(posedge clk or negedge rst_n) begin : seq_logic
if (!rst_n) begin
current_state <= IDLE;
done <= 0;
error <= 0;
// Initialize others...
end else begin
current_state <= next_state;
unique case(next_state)
IDLE: {done,error} <= 'b0;
RECEIVE_FLAG: /* update based on detection results */
// ...
COLLECT_DATA: /* accumulate until full word assembled */
// ...
CHECK_CRC: /* set final outcome indicators accordingly */
// ...
ERROR_STATE: {done,error} <= 'b10; // Error condition signaled
default:;
endcase
end
end
```
阅读全文
相关推荐

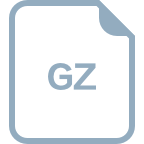















