keras_multi_head 在keras那个版本中
时间: 2023-07-09 14:15:31 浏览: 76
keras_multi_head 库是为 Keras 2.2.4 及更高版本开发的。如果您使用的是旧版本的 Keras,则可能会遇到问题。建议您在使用 keras_multi_head 之前,将 Keras 更新到最新版本。您可以使用以下命令升级 Keras:
```
pip install --upgrade keras
```
请注意,如果您使用的是 TensorFlow 2.0 或更高版本,则无需安装 Keras,因为 Keras 已经作为 TensorFlow 的一部分被集成了。
相关问题
keras_multi_head 的MultiheadAttention的参数
Keras的MultiheadAttention层的参数包括:
- `num_heads`:`int`类型,表示多头注意力机制中的头数(注意力子空间的数量)。默认值为8。
- `head_size`:`int`类型,表示每个头的向量维度。默认值为`None`,表示自动计算为`model_dim // num_heads`。
- `dropout`:`float`类型,表示对注意力权重进行dropout的比率。默认值为0。
- `use_bias`:`bool`类型,表示是否使用偏置向量。默认值为`True`。
- `return_attention_scores`:`bool`类型,表示是否返回注意力权重。默认值为`False`。
除此之外,该层还有一个可选参数`output_shape`,用于指定输出形状。默认值为`None`,表示输出形状与输入形状相同。
keras实现multi-head self-attention代码
### 回答1:
以下是使用 Keras 实现 Multi-Head Self-Attention 的代码示例:
```python
from tensorflow import keras
from tensorflow.keras import layers
class MultiHeadSelfAttention(layers.Layer):
def __init__(self, embed_dim, num_heads=8):
super(MultiHeadSelfAttention, self).__init__()
self.embed_dim = embed_dim
self.num_heads = num_heads
if embed_dim % num_heads != 0:
raise ValueError(
f"embed_dim ({embed_dim}) must be divisible by num_heads ({num_heads})"
)
self.projection_dim = embed_dim // num_heads
self.query_dense = layers.Dense(embed_dim)
self.key_dense = layers.Dense(embed_dim)
self.value_dense = layers.Dense(embed_dim)
self.combine_heads = layers.Dense(embed_dim)
def attention(self, query, key, value):
score = tf.matmul(query, key, transpose_b=True)
dim_key = tf.cast(tf.shape(key)[-1], tf.float32)
scaled_score = score / tf.math.sqrt(dim_key)
weights = tf.nn.softmax(scaled_score, axis=-1)
output = tf.matmul(weights, value)
return output, weights
def separate_heads(self, x, batch_size):
x = tf.reshape(x, (batch_size, -1, self.num_heads, self.projection_dim))
return tf.transpose(x, perm=[0, 2, 1, 3])
def call(self, inputs):
# x.shape = [batch_size, seq_len, embedding_dim]
batch_size = tf.shape(inputs)[0]
query = self.query_dense(inputs) # (batch_size, seq_len, embed_dim)
key = self.key_dense(inputs) # (batch_size, seq_len, embed_dim)
value = self.value_dense(inputs) # (batch_size, seq_len, embed_dim)
query = self.separate_heads(
query, batch_size
) # (batch_size, num_heads, seq_len, projection_dim)
key = self.separate_heads(
key, batch_size
) # (batch_size, num_heads, seq_len, projection_dim)
value = self.separate_heads(
value, batch_size
) # (batch_size, num_heads, seq_len, projection_dim)
attention, weights = self.attention(query, key, value)
attention = tf.transpose(
attention, perm=[0, 2, 1, 3]
) # (batch_size, seq_len, num_heads, projection_dim)
concat_attention = tf.reshape(
attention, (batch_size, -1, self.embed_dim)
) # (batch_size, seq_len, embed_dim)
output = self.combine_heads(
concat_attention
) # (batch_size, seq_len, embed_dim)
return output
```
在上面的代码中,我们定义了一个名为 `MultiHeadSelfAttention` 的自定义 Keras 层。在 `__init__` 方法中,我们定义了以下变量:
- `embed_dim`:嵌入维度。
- `num_heads`:头的数量。
- `projection_dim`:每个头的投影维度。
- `query_dense`、`key_dense` 和 `value_dense`:三个全连接层,用于将输入嵌入到 `embed_dim` 维空间中。
- `combine_heads`:全连接层,用于将多头注意力的输出组合成一个 `embed_dim` 维张量。
在 `call` 方法中,我们首先使用 `query_dense`、`key_dense` 和 `value_dense` 将输入嵌入到 `embed_dim` 维空间中。然后,我们将查询、键和值分别投影到 `num_heads` 个子空间中,并计算每个子空间的注意力输出。最后,我们将 `num_heads` 个子空间的注意力输出组合成一个 `embed_dim` 维张量,并通过 `combine_heads` 层进行组合。
### 回答2:
Keras是一个流行的深度学习库,它提供了方便的API来实现各种神经网络模型。其中,多头自注意力(multi-head self-attention)是一种在自然语言处理中广泛使用的技术,可以用于提取输入序列之间的重要关系。
下面是使用Keras实现多头自注意力的代码示例:
```python
import tensorflow.keras as keras
from keras.layers import Layer, Dense
class MultiHeadSelfAttention(Layer):
def __init__(self, n_heads, d_model, **kwargs):
super(MultiHeadSelfAttention, self).__init__(**kwargs)
self.n_heads = n_heads
self.d_model = d_model
self.wq = Dense(d_model)
self.wk = Dense(d_model)
self.wv = Dense(d_model)
self.dense = Dense(d_model)
def call(self, inputs):
q = self.wq(inputs)
k = self.wk(inputs)
v = self.wv(inputs)
q = self.split_heads(q)
k = self.split_heads(k)
v = self.split_heads(v)
attention_weights = keras.layers.dot([q, k], axes=[-1, -1])
attention_weights = keras.layers.Activation('softmax')(attention_weights)
output = keras.layers.dot([attention_weights, v], axes=[-1, 1])
output = self.combine_heads(output)
output = self.dense(output)
return output
def split_heads(self, x):
batch_size = keras.backend.shape(x)[0]
seq_length = keras.backend.shape(x)[1]
d_model = self.d_model
split_size = d_model // self.n_heads
x = keras.backend.reshape(x, (batch_size, seq_length, self.n_heads, split_size))
return keras.backend.permute_dimensions(x, (0, 2, 1, 3))
def combine_heads(self, x):
batch_size = keras.backend.shape(x)[0]
seq_length = keras.backend.shape(x)[2]
d_model = self.d_model
x = keras.backend.permute_dimensions(x, (0, 2, 1, 3))
return keras.backend.reshape(x, (batch_size, seq_length, d_model))
```
上述代码中,我们创建了一个名为MultiHeadSelfAttention的自定义层,它继承自Keras的Layer类。在构造函数中,我们指定了注意力头数n_heads和模型维度d_model。在call函数中,我们分别通过全连接层将输入序列映射为查询(q)、键(k)和值(v)的表示。然后,我们将这些表示进行头分割,计算注意力权重,并应用这些权重来聚合值。最后,我们通过全连接层将聚合后的结果映射回原始维度。
通过使用上述代码示例,我们可以在Keras中轻松实现多头自注意力机制,并将其用于自然语言处理等任务中。
### 回答3:
Keras是一个流行的深度学习框架,可以用于实现各种神经网络模型,包括self-attention模型。Multi-head self-attention是一种扩展的self-attention模型,用于加强模型对输入数据中不同部分的关注能力。
具体实现multi-head self-attention模型的代码如下:
1. 引入所需的Keras库和模块:
```python
from tensorflow import keras
from tensorflow.keras.layers import Dense, Input, Dropout, LayerNormalization
from tensorflow.keras import Model
```
2. 定义multi-head self-attention层的类:
```python
class MultiHeadSelfAttention(keras.layers.Layer):
def __init__(self, d_model, num_heads):
super(MultiHeadSelfAttention, self).__init__()
self.num_heads = num_heads
self.d_model = d_model
self.depth = int(d_model / num_heads)
self.query_dense = Dense(d_model)
self.key_dense = Dense(d_model)
self.value_dense = Dense(d_model)
self.dense = Dense(d_model)
def split_heads(self, x, batch_size):
x = keras.backend.reshape(x, (batch_size, -1, self.num_heads, self.depth))
return keras.backend.transpose(x, perm=[0, 2, 1, 3])
def call(self, inputs):
query = inputs
key = inputs
value = inputs
batch_size = keras.backend.shape(query)[0]
query = self.query_dense(query)
key = self.key_dense(key)
value = self.value_dense(value)
query = self.split_heads(query, batch_size)
key = self.split_heads(key, batch_size)
value = self.split_heads(value, batch_size)
scaled_attention_outputs, attention_weights = self.compute_attention(query, key, value)
scaled_attention = keras.backend.transpose(scaled_attention_outputs, perm=[0, 2, 1, 3])
concat_attention = keras.backend.reshape(scaled_attention, (batch_size, -1, self.d_model))
outputs = self.dense(concat_attention)
return outputs, attention_weights
def compute_attention(self, query, key, value):
matmul_qk = keras.backend.batch_dot(query, key, axes=[-1, -1])
scaled_attention_logits = matmul_qk / keras.backend.sqrt(keras.backend.cast(self.depth, dtype=keras.backend.floatx()))
attention_weights = keras.backend.softmax(scaled_attention_logits)
attention_outputs = keras.backend.batch_dot(attention_weights, value, axes=[-1, 2])
return attention_outputs, attention_weights
```
3. 构建完整的模型:
```python
def create_model(d_model=256, num_heads=8):
inputs = Input(shape=(seq_length, d_model))
attention_layer = MultiHeadSelfAttention(d_model, num_heads)
attention_outputs, attention_weights = attention_layer(inputs)
dropout = Dropout(0.1)(attention_outputs)
normalization = LayerNormalization(epsilon=1e-6)(dropout)
dense = Dense(d_model, activation='relu')(normalization)
outputs = Dense(num_classes, activation='softmax')(dense)
model = Model(inputs=inputs, outputs=outputs)
return model
```
这段代码实现了一个包含multi-head self-attention层的完整模型,输入shape为(seq_length, d_model),输出为一个softmax分类器的结果。考虑到不同应用场景下的具体要求,可以根据实际需要自定义模型的层数、宽度以及其他配置,来构建一个更适合具体任务的multi-head self-attention模型。
相关推荐
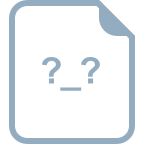
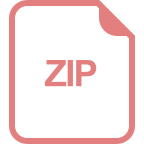
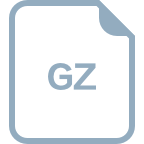












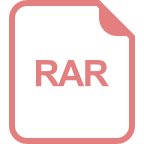