编写Person类,包含人的姓名和年龄;编写自定义异常类IllegalAgeException,当用户输入的年龄小于0的时候,抛出IllegalAgeException异常,为用户友好提示错误信息。 测试类代码如下: public class TestPersonException { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); try { Person p1 = new Person(scanner.next(), scanner.nextInt()); System.out.println(p1.toString()); } catch (IllegalAgeException e) { System.out.println(e.getMessage()); System.out.println("你输入的年龄为" + e.getAge()); } } }
时间: 2024-02-14 15:10:57 浏览: 25
好的,我理解了你的问题。以下是代码实现:
```
public class Person {
private String name;
private int age;
public Person(String name, int age) throws IllegalAgeException {
if (age < 0) {
throw new IllegalAgeException("年龄不能小于0!");
}
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
public class IllegalAgeException extends Exception {
private int age;
public IllegalAgeException(String message) {
super(message);
}
public IllegalAgeException(String message, int age) {
super(message);
this.age = age;
}
public int getAge() {
return age;
}
}
public class TestPersonException {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
try {
Person p1 = new Person(scanner.next(), scanner.nextInt());
System.out.println(p1.toString());
} catch (IllegalAgeException e) {
System.out.println(e.getMessage());
System.out.println("你输入的年龄为" + e.getAge());
}
}
}
```
在Person类的构造函数中,如果年龄小于0,则抛出IllegalAgeException异常,并且传递友好提示信息到异常类中。在测试类中,我们通过try-catch语句捕获异常,并且输出友好提示信息和用户输入的年龄。
相关推荐
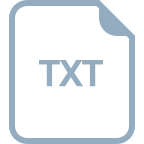
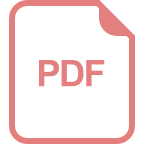














