自定义非法年龄类IllegalAgeException,定义一个Person类,包含年龄,姓名,性别等属性,编写属性设置和读取函数,在设置年龄函数中,判断参数是否符合要求(1~150),如果不符合则抛出异常,编写main函数进行测试。Java实现
时间: 2023-08-31 10:41:29 浏览: 132
### 回答1:
在 Java 中,可以通过定义自定义异常类来实现自定义异常。下面是如何定义 `IllegalAgeException` 异常类和 `Person` 类的代码示例:
```java
// 自定义非法年龄异常类
class IllegalAgeException extends Exception {
public IllegalAgeException(String message) {
super(message);
}
}
// Person 类
class Person {
private int age;
private String name;
private String gender;
// 年龄属性的设置函数
public void setAge(int age) throws IllegalAgeException {
if (age < 1 || age > 150) {
throw new IllegalAgeException("非法的年龄: " + age);
}
this.age = age;
}
// 年龄属性的读取函数
public int getAge() {
return this.age;
}
// 姓名属性的设置函数
public void setName(String name) {
this.name = name;
}
// 姓名属性的读取函数
public String getName() {
return this.name;
}
// 性别属性的设置函数
public void setGender(String gender) {
this.gender = gender;
}
// 性别属性的读取函数
public String getGender() {
return this.gender;
}
}
```
下面是如何在 `main` 函数中测试这两个类的代码示例:
```java
public static void main(String[] args) {
Person person = new Person();
try {
// 设置年龄为 200,应抛出非法年龄异常
person.setAge(200);
} catch (IllegalAgeException e) {
System.out.println(e.getMessage());
}
try {
// 设置年龄为 20,应设置成功
person.setAge(20);
System.out.println("年龄设置成功: " + person.getAge());
} catch (IllegalAgeException e) {
System.out.println(e.getMessage());
}
}
```
在上
### 回答2:
自定义非法年龄类IllegalAgeException,可以继承自Java中的Exception类。在该类中,我们可以定义一个构造函数,用于接收异常信息,并调用父类的构造函数将设置的错误信息传递给父类。例如:
```java
public class IllegalAgeException extends Exception {
public IllegalAgeException(String message) {
super(message);
}
}
```
然后,我们可以定义Person类,包含年龄、姓名、性别等属性,以及相应的读取和设置函数:
```java
public class Person {
private int age;
private String name;
private String gender;
public int getAge() {
return age;
}
public void setAge(int age) throws IllegalAgeException {
if (age < 1 || age > 150) {
throw new IllegalAgeException("年龄必须在1~150之间");
}
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
```
最后,在main函数中进行测试:
```java
public class Main {
public static void main(String[] args) {
Person person = new Person();
try {
person.setAge(18);
person.setName("张三");
person.setGender("男");
System.out.println("姓名:" + person.getName() + ",年龄:" + person.getAge() + ",性别:" + person.getGender());
} catch (IllegalAgeException e) {
System.out.println(e.getMessage());
}
}
}
```
以上代码中,我们通过setAge()函数设置了一个合法的年龄18,然后分别设置了姓名和性别,最后通过get函数获取属性值,并打印输出。如果年龄不符合要求,则会抛出IllegalAgeException异常,我们在catch块中捕获并输出异常信息。
### 回答3:
自定义非法年龄类IllegalAgeException,继承自Exception类,通过重新实现构造函数来设置异常信息。在构造函数中,传入一个String类型的参数message,用于设置异常信息。
```java
public class IllegalAgeException extends Exception {
public IllegalAgeException(String message) {
super(message);
}
}
```
定义一个Person类,包含年龄,姓名,性别等属性。通过使用private修饰符来确保数据的封装性,提供相应的公共方法来设置和读取属性。
```java
public class Person {
private int age;
private String name;
private String gender;
public int getAge() {
return age;
}
public void setAge(int age) throws IllegalAgeException {
if (age < 1 || age > 150) {
throw new IllegalAgeException("年龄不合法,必须在1~150之间。");
}
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
```
编写main函数进行测试。
```java
public class Main {
public static void main(String[] args) {
Person person = new Person();
try {
person.setAge(20);
person.setName("张三");
person.setGender("男");
System.out.println("姓名:" + person.getName() + ",年龄:" + person.getAge() + ",性别:" + person.getGender());
} catch (IllegalAgeException e) {
System.out.println(e.getMessage()); // 输出年龄不合法的提示信息
}
}
}
```
阅读全文
相关推荐
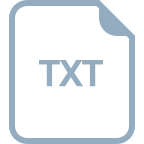
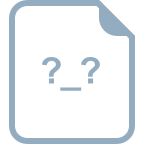



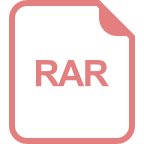
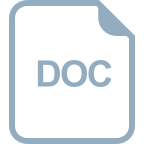
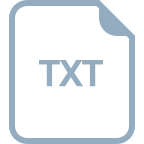
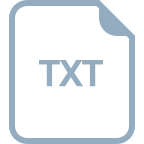
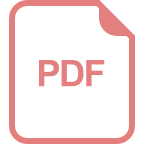
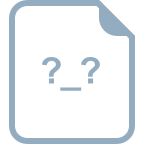
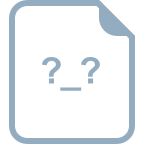
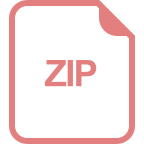
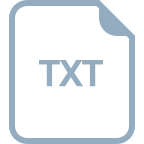
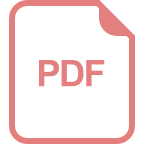
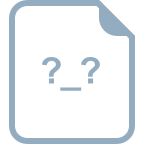
