c语言编辑框
时间: 2023-07-02 17:21:16 浏览: 110
为了在C语言中创建编辑框,你可以使用Windows API中的CreateWindow函数和Edit类。以下是一个简单示例:
```c
#include <windows.h>
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
// Register the window class.
const char CLASS_NAME[] = "MyClass";
WNDCLASS wc = { };
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
// Create the window.
HWND hwnd = CreateWindowEx(
0, // Optional window styles.
CLASS_NAME, // Window class
"C语言编辑框", // Window text
WS_OVERLAPPEDWINDOW, // Window style
// Size and position
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, // Parent window
NULL, // Menu
hInstance, // Instance handle
NULL // Additional application data
);
if (hwnd == NULL)
{
return 0;
}
ShowWindow(hwnd, nCmdShow);
// Run the message loop.
MSG msg = { };
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
// Handle other messages.
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
```
这将创建一个空白窗口。要在该窗口中添加编辑框,请在WndProc函数中添加以下代码:
```c
case WM_CREATE:
{
HWND hwndEdit = CreateWindowEx(
WS_EX_CLIENTEDGE, // Optional styles
"EDIT", // Edit class
"", // Text
WS_CHILD | WS_VISIBLE | ES_MULTILINE | ES_AUTOVSCROLL | ES_AUTOHSCROLL,
0, 0, 100, 100, // Position and size
hwnd, // Parent window
NULL, // No menu
GetModuleHandle(NULL), // Instance handle
NULL // Pointer to extra data
);
if (hwndEdit == NULL)
{
MessageBox(hwnd, "Could not create edit box.", "Error", MB_OK | MB_ICONERROR);
}
}
break;
```
这将在窗口中创建一个具有多行文本编辑功能的编辑框。你可以在CreateWindowEx函数中通过修改样式和位置来调整编辑框的大小和外观。
阅读全文
相关推荐


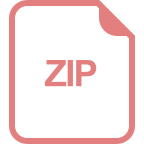

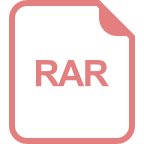
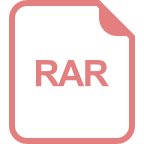





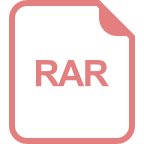





