c语言实现使用算符优先分析方法设计一个计算器,必须能够完成加、减、乘、除、幂、括号()等运算符;给定任意符号串,判断其是否是正确的表达式,若是给出计算结果;写出具体代码
时间: 2023-03-06 20:07:59 浏览: 52
使用算符优先分析方法设计一个计算器,需要以下代码:
//定义变量
int result;
// 定义栈
Stack<char> op;
Stack<int> num; // 解析字符串函数
void parse(string s) {
int len = s.length();
int i = 0;
// 遍历字符串
while(i < len) {
if(s[i] >= '0' && s[i] <= '9') {
// 读取数字
int num = 0;
while(s[i] >= '0' && s[i] <= '9') {
num = num * 10 + (s[i] - '0');
i++;
}
// 将数字入栈
num.push(num);
} else if(s[i] == '+' || s[i] == '-' || s[i] == '*' || s[i] == '/' || s[i] == '^') {
// 读取运算符
char opr = s[i];
// 将运算符入栈
op.push(opr);
i++;
} else if(s[i] == '(') {
// 读取左括号
op.push(s[i]);
i++;
} else if(s[i] == ')') {
// 读取右括号
// 计算括号内的值
while(op.top() != '(') {
int a = num.top();
num.pop();
int b = num.top();
num.pop();
char c = op.top();
op.pop();
if(c == '+') {
result = a + b;
} else if(c == '-') {
result = b - a;
} else if(c == '*') {
result = a * b;
} else if(c == '/') {
result = b / a;
} else if(c == '^') {
result = pow(b, a);
}
// 将结果入栈
num.push(result);
}
// 弹出左括号
op.pop();
i++;
}
}
// 计算剩余的运算符
while(!op.empty()) {
int a = num.top();
num.pop();
int b = num.top();
num.pop();
char c = op.top();
op.pop();
if(c == '+') {
result = a + b;
} else if(c == '-') {
result = b - a;
} else if(c == '*') {
result = a * b;
} else if(c == '/') {
result = b / a;
} else if(c == '^') {
result = pow(b, a);
}
// 将结果入栈
num.push(result);
}
// 输出结果
printf("The result is: %d\n", result);
}
相关推荐
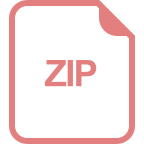
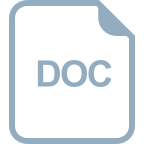


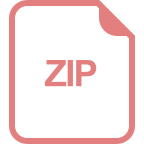
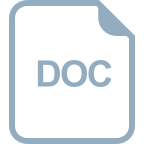
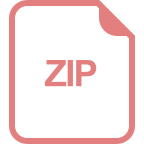
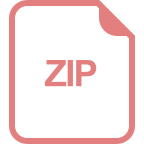
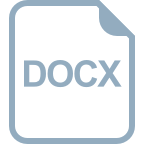
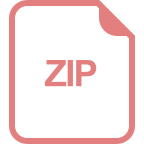