python turtle 用stamp()实现贪吃蛇的程序
时间: 2024-05-06 12:20:59 浏览: 88
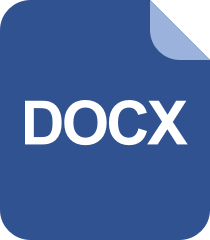
在 Python 中使用 Turtle 创建贪吃蛇游戏
好的,我可以回答这个问题。你可以参考以下代码实现:
```python
import turtle
import random
# set up the screen
window = turtle.Screen()
window.title("Snake Game")
window.bgcolor("white")
window.setup(width=600, height=600)
# create the snake
snake = []
for i in range(3):
square = turtle.Turtle()
square.shape("square")
square.color("black")
square.penup()
square.goto(i*-20, 0)
snake.append(square)
# create the food
food = turtle.Turtle()
food.shape("circle")
food.color("red")
food.penup()
food.goto(random.randint(-290, 290), random.randint(-290, 290))
# set up the movement
direction = "stop"
def up():
global direction
direction = "up"
def down():
global direction
direction = "down"
def left():
global direction
direction = "left"
def right():
global direction
direction = "right"
window.listen()
window.onkeypress(up, "w")
window.onkeypress(down, "s")
window.onkeypress(left, "a")
window.onkeypress(right, "d")
# set up the game loop
while True:
window.update()
# move the snake
for i in range(len(snake)-1, 0, -1):
x = snake[i-1].xcor()
y = snake[i-1].ycor()
snake[i].goto(x, y)
if len(snake) > 0:
x = snake[0].xcor()
y = snake[0].ycor()
if direction == "up":
snake[0].sety(y + 20)
elif direction == "down":
snake[0].sety(y - 20)
elif direction == "left":
snake[0].setx(x - 20)
elif direction == "right":
snake[0].setx(x + 20)
# check for collision with food
if snake[0].distance(food) < 20:
food.goto(random.randint(-290, 290), random.randint(-290, 290))
square = turtle.Turtle()
square.shape("square")
square.color("black")
square.penup()
snake.append(square)
# check for collision with walls
if snake[0].xcor() < -290 or snake[0].xcor() > 290 or snake[0].ycor() < -290 or snake[0].ycor() > 290:
break
# check for collision with body
for square in snake[1:]:
if snake[0].distance(square) < 10:
break
# use stamp() to display the snake
for i, square in enumerate(snake):
square.clearstamps()
square.setpos(i*-20, 0)
square.stamp()
# game over
game_over = turtle.Turtle()
game_over.hideturtle()
game_over.color("red")
game_over.write("Game Over", align="center", font=("Arial", 24, "bold"))
```
这个程序使用turtle模块实现了贪吃蛇游戏,其中使用了stamp()方法来在屏幕上显示蛇的身体。注意,这只是一个简单的实现,你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
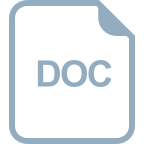
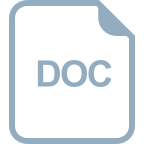


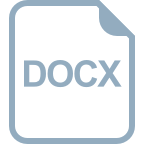
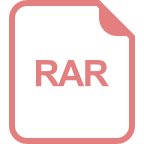
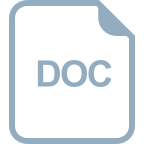
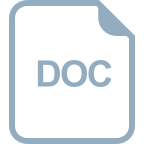


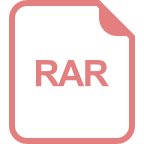
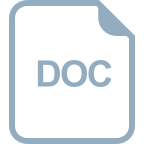


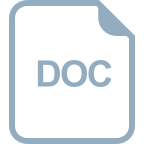
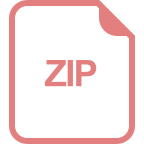
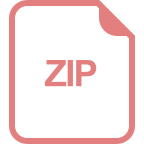
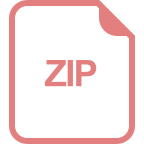