subprocess.Popen和run
时间: 2023-12-09 09:32:18 浏览: 361
subprocess.Popen和subprocess.run都是Python中用于执行外部命令的模块。它们的主要区别在于Popen是异步执行,而run是同步执行。
具体来说,Popen会立即返回一个Popen对象,然后在后台异步执行命令。而run则会一直等待命令执行完成,然后返回一个CompletedProcess对象,其中包含了命令的执行结果。
下面是一个使用Popen执行命令的例子:
```python
import subprocess
p = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = p.communicate()
print(stdout.decode())
```
这个例子执行了一个ls -l命令,并将结果输出到stdout变量中。注意,我们使用了communicate()方法来等待命令执行完成,并获取命令的输出结果。
下面是一个使用run执行命令的例子:
```python
import subprocess
result = subprocess.run(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
print(result.stdout.decode())
```
这个例子同样执行了一个ls -l命令,并将结果输出到stdout变量中。不同的是,我们使用了run方法来同步执行命令,并获取命令的输出结果。
相关问题
subprocess.Popen subprocess.run
subprocess.Popen and subprocess.run are both modules in Python's subprocess module that are used for running external commands. Here's a brief explanation of each:
1. subprocess.Popen: This function is used to create a new process and execute a command within it. It provides more flexibility and control over the execution process. Popen returns a Popen object, which allows you to interact with the running process, such as reading its output or terminating it.
Example usage:
```python
import subprocess
# Run a command and get its output
result = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE)
output = result.communicate()
print(output.decode('utf-8'))
```
2. subprocess.run: This function is a higher-level convenience function introduced in Python 3.5. It is used to run a command and wait for it to complete. It returns a CompletedProcess object that provides information about the completed process, such as return code and output.
Example usage:
```python
import subprocess
# Run a command and get its output
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
```
Both functions can be used to execute external commands, but the choice between them depends on your requirements. Popen offers more flexibility, while run provides a simpler and more straightforward way to execute commands.
subprocess.run和subprocess.Popen的区别
`subprocess.run`和`subprocess.Popen`是Python的两个用于执行外部命令的函数,它们之间有一些区别。
`subprocess.run`是Python 3.5及更高版本引入的函数,它是一个高级接口,使用起来更加简单方便。它会等待子进程完成,并返回一个`CompletedProcess`对象,其中包含了运行结果的相关信息,如返回码、标准输出和标准错误等。下面是`subprocess.run`的一个例子:
```python
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.returncode)
print(result.stdout)
print(result.stderr)
```
`subprocess.Popen`是一个低级接口,可以更加灵活地控制子进程。它返回一个`Popen`对象,可以通过该对象的方法和属性来与子进程进行交互。需要手动调用`Popen.wait()`等待子进程完成,并通过`Popen.communicate()`方法获取子进程的输出。下面是`subprocess.Popen`的一个例子:
```python
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
returncode = process.returncode
print(returncode)
print(stdout)
print(stderr)
```
总结来说,`subprocess.run`是一种更简单易用的接口,适用于大多数外部命令执行的场景;而`subprocess.Popen`则提供了更多的灵活性和控制权,适用于一些需要更细粒度控制的情况。
阅读全文
相关推荐
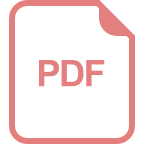
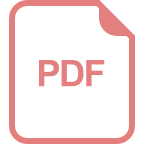
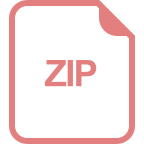














