import os import subprocess class ControlLd: def __init__(self,path): os.putenv('Path',path) print(os.putenv('Path',path)) def run_command(self,command): """运行cmd命令""" pipe=subprocess.Popen(args=command,stdout=subprocess.PIPE,shell=True,encoding='gbk') pipe=pipe.stdout.read() return pipe
时间: 2024-01-26 14:03:39 浏览: 178
这是一个 Python 类 ControlLd,它的作用是运行 cmd 命令。它有两个方法:
1. `__init__(self, path)` 方法:初始化类,并将系统环境变量 Path 设置为 path。
2. `run_command(self, command)` 方法:运行 cmd 命令,返回命令输出。
在 `run_command()` 方法中,首先使用 `subprocess.Popen()` 函数创建一个子进程,执行传入的命令。`stdout=subprocess.PIPE` 表示将子进程的输出重定向到一个管道中,`shell=True` 表示在命令行中执行命令。使用 `encoding='gbk'` 将输出编码设置为 GBK,以便在 Windows 系统上正确显示中文字符。
最后,使用 `stdout.read()` 方法读取管道中的输出,并将其返回。
相关问题
import tkinter as tkfrom tkinter import filedialogimport subprocessclass GUI(tk.Frame): def __init__(self, master=None): super().__init__(master) self.master = master self.master.title("软件界面") self.pack() self.path_label = tk.Label(self, text="输入路径:") self.path_label.pack() self.path_entry = tk.Entry(self) self.path_entry.pack() self.path_name_label = tk.Label(self, text="输入路径名:") self.path_name_label.pack() self.path_name_entry = tk.Entry(self) self.path_name_entry.pack() self.run_button = tk.Button(self, text="运行", command=self.run_program) self.run_button.pack() self.output_label = tk.Label(self, text="输出:") self.output_label.pack() self.output_text = tk.Text(self) self.output_text.pack() def run_program(self): path = self.path_entry.get() path_name = self.path_name_entry.get() cmd = ['python', 'yourprogram.py', path, path_name] result = subprocess.run(cmd, capture_output=True) self.output_text.insert(tk.END, result.stdout.decode())if __name__ == "__main__": root = tk.Tk() app = GUI(master=root) app.mainloop() 和import sysif __name__ == "__main__": # 获取从命令行传递过来的参数 path = sys.argv[1] path_name = sys.argv[2] # 在这里写你的程序逻辑 # ...这2个代码怎么关联不上
这两段代码是用来实现从界面输入路径和路径名,然后调用命令行运行Python程序的。具体来说,第一段代码是使用tkinter库创建了一个GUI界面,包括两个输入框、一个运行按钮和一个输出框。当用户点击运行按钮时,会获取输入框中的路径和路径名,并将其作为参数调用subprocess库运行Python程序。
而第二段代码则是一个独立的Python程序,它使用sys库获取命令行传递的参数,然后根据路径和路径名执行相应的程序逻辑。这段代码需要在第一段代码中调用,使用subprocess库运行该Python程序,并传递路径和路径名作为参数。具体来说,第一段代码的cmd变量中的'yourprogram.py'应该替换为第二段代码的Python程序文件名。
import queue import threading import cv2 as cv import subprocess as sp class Live(object): def __init__(self): self.frame_queue = queue.Queue() self.command = "" # 自行设置 self.rtmpUrl = "" self.camera_path = "" def read_frame(self): print("开启推流") cap = cv.VideoCapture(self.camera_path) # Get video information fps = int(cap.get(cv.CAP_PROP_FPS)) width = int(cap.get(cv.CAP_PROP_FRAME_WIDTH)) height = int(cap.get(cv.CAP_PROP_FRAME_HEIGHT)) # ffmpeg command self.command = ['ffmpeg', '-y', '-f', 'rawvideo', '-vcodec','rawvideo', '-pix_fmt', 'bgr24', '-s', "{}x{}".format(width, height), '-r', str(fps), '-i', '-', '-c:v', 'libx264', '-pix_fmt', 'yuv420p', '-preset', 'ultrafast', '-f', 'flv', self.rtmpUrl] # read webcamera while(cap.isOpened()): ret, frame = cap.read() if not ret: print("Opening camera is failed") break # put frame into queue self.frame_queue.put(frame) def push_frame(self): # 防止多线程时 command 未被设置 while True: if len(self.command) > 0: # 管道配置 p = sp.Popen(self.command, stdin=sp.PIPE) break while True: if self.frame_queue.empty() != True: frame = self.frame_queue.get() # process frame # 你处理图片的代码 # write to pipe p.stdin.write(frame.tostring()) def run(self): threads = [ threading.Thread(target=Live.read_frame, args=(self,)), threading.Thread(target=Live.push_frame, args=(self,)) ] [thread.setDaemon(True) for thread in threads] [thread.start() for thread in threads]
这是一个 Python 类,用于推送摄像头视频流到 RTMP 服务器。该类包含以下方法:
1. `__init__(self)`:初始化方法,设置摄像头路径、RTMP 推流地址和帧队列。
2. `read_frame(self)`:读取摄像头视频流,将视频帧存入帧队列中。
3. `push_frame(self)`:从帧队列中取出视频帧,进行处理后写入到管道中,最终推送到 RTMP 服务器。
4. `run(self)`:开启两个线程,分别执行 `read_frame` 和 `push_frame` 方法。
为了正常运行该类,需要安装 `queue`、`threading`、`cv2` 和 `subprocess` 模块。同时需要在初始化方法中设置 `rtmpUrl` 和 `camera_path` 两个参数,分别为 RTMP 推流地址和摄像头路径。在 `push_frame` 方法中需要根据实际需求对视频帧进行处理。
阅读全文
相关推荐
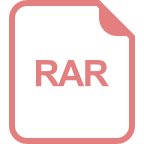
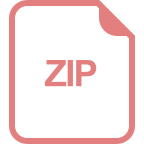
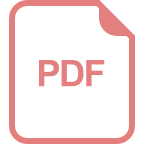












