在多线程间 如何通过 std::promise 返回一个std::any
时间: 2023-08-02 07:11:37 浏览: 56
在多线程环境下,使用 `std::promise` 返回 `std::any` 时需要注意线程安全。可以使用 `std::shared_ptr` 来确保多个线程可以访问同一个 `std::promise` 和 `std::future` 对象。
例如,可以创建一个 `std::shared_ptr`,并将其传递给多个线程。每个线程都可以通过该 `std::shared_ptr` 访问 `std::promise` 和 `std::future` 对象,然后在 `std::promise` 中设置值,最后在 `std::future` 中获取值。
下面是一个示例代码:
```c++
#include <iostream>
#include <thread>
#include <future>
#include <any>
class MyAny {
public:
MyAny(std::any value) : value_(std::move(value)) {}
std::any getValue() { return value_; }
private:
std::any value_;
};
void worker(std::shared_ptr<std::promise<MyAny>> p) {
std::any value = 42;
MyAny myValue(value);
p->set_value(myValue);
}
int main() {
std::shared_ptr<std::promise<MyAny>> p = std::make_shared<std::promise<MyAny>>();
std::future<MyAny> f = p->get_future();
std::thread t(worker, p);
MyAny result = f.get();
std::any resultValue = result.getValue();
std::cout << std::any_cast<int>(resultValue) << std::endl;
t.join();
return 0;
}
```
在上面的示例代码中,我们首先创建一个 `std::shared_ptr`,然后将其传递给一个线程和 `std::promise` 对象。线程可以通过该 `std::shared_ptr` 访问 `std::promise` 对象,并在其中设置一个值。主线程可以通过 `std::future` 对象获取该值,并通过 `MyAny` 类的方法获取 `std::any` 类型的值。需要注意的是,在主线程中获取值之前,必须确保线程已经完成并设置了该值。在本例中,我们使用 `std::thread::join` 等待线程完成。
相关推荐
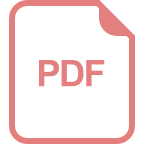
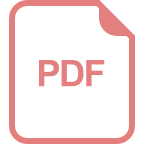
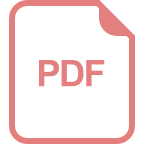














