输入一个正整数repeat (0 < repeat < 10),做repeat 次下列运算: 先输入一个正整数n, 接下来再输入n 个整数,然后从这n个数中输出最大值。
时间: 2024-11-27 22:16:50 浏览: 139
这个程序要求你编写一个简单的迭代过程,让用户反复输入一组数字并找到其中的最大值,直到指定重复次数(repeat)结束。下面是实现该功能的C语言代码示例:
```c
#include <stdio.h>
int main() {
int repeat;
// 获取重复次数
printf("Enter the number of times to repeat the operation (0 < repeat < 10): ");
scanf("%d", &repeat);
while (repeat < 0 || repeat >= 10) {
printf("Invalid input. Please enter a positive integer less than 10: ");
scanf("%d", &repeat);
}
for (int i = 0; i < repeat; i++) {
int n;
// 输入数组大小
printf("Enter the number of integers for this iteration: ");
scanf("%d", &n);
if (n <= 0) {
printf("Invalid input. Please enter a positive integer: ");
continue;
}
int* numbers = malloc(n * sizeof(int)); // 动态分配内存
if (!numbers) {
perror("Memory allocation failed");
return 1;
}
// 输入并找出最大值
int max_value = -1;
for (int j = 0; j < n; j++) {
scanf("%d", &numbers[j]);
if (numbers[j] > max_value) {
max_value = numbers[j];
}
}
// 输出最大值
printf("The maximum value is: %d\n", max_value);
free(numbers); // 释放内存
}
return 0;
}
```
在这个程序中,我们首先获取用户的重复次数,如果不符合条件则提示重新输入。接着在循环内,每次请求用户输入数组的大小`n`,并读取`n`个整数。我们动态地为这些数字分配内存,然后遍历数组找到最大值并打印出来。最后别忘了在循环结束后释放内存。
阅读全文
相关推荐
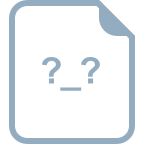
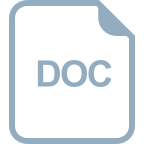















