建立一个存储字符的线性表,从键盘输入线性表中元素,如果输入为“@”,则中止输入。(用C语言链表编写)
时间: 2024-11-03 11:22:32 浏览: 20
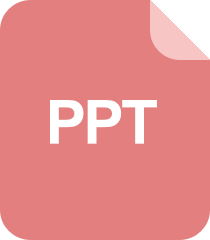
链表L(带头结点)有个元素-数据结构线性表
在C语言中,我们可以使用结构体来表示字符,并通过单向链表来存储这个线性表。首先,我们需要定义一个结构体`Node`来包含字符和指向下一个节点的指针:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
char data;
struct Node* next;
} Node;
// 创建新节点并插入链表
Node* createNode(char ch) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode) {
newNode->data = ch;
newNode->next = NULL;
}
return newNode;
}
int main() {
Node* head = NULL; // 链表头指针
Node* current = NULL; // 当前节点
while (1) { // 循环直到遇到终止符@
char input;
printf("请输入字符(输入 '@' 结束):");
scanf("%c", &input);
if (input == '@') {
break; // 输入 @ 时跳出循环
}
Node* newNode = createNode(input);
if (head == NULL) {
head = newNode;
current = head;
} else {
current->next = newNode;
current = newNode;
}
}
printf("输入的字符线性表:");
Node* temp = head;
while (temp != NULL) {
printf("%c -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
return 0;
}
```
在这个程序中,我们创建了一个名为`createNode`的函数用于生成新的链表节点,然后在主循环中持续读取用户输入,每当输入非`@`字符时就添加到链表中。当用户输入`@`时,跳出循环并打印出整个链表。
阅读全文
相关推荐
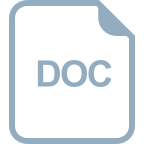
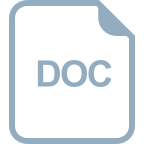
















