创建链表并读入图书信息。 计算所有图书的平均价格,并调整价格。 查找价格最高的图书。 插入新图书到指定位置。 删除指定位置的旧图书。使用c语言写代码
时间: 2024-11-15 22:24:20 浏览: 15
在C语言中,我们可以创建一个简单的链表结构来存储图书信息。这里是一个基本的例子,包括链表操作:
首先,我们需要定义一个结构体来表示一本书,包含书名、作者和价格:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Book {
char title[50];
char author[50];
float price;
struct Book* next; // 指向下一个节点
} Book;
```
接下来,我们实现链表的基本操作:
1. **创建链表**:
```c
Book* createList() {
Book* head = NULL;
return head;
}
void insert(Book** head, char* title, char* author, float price) {
Book* newNode = (Book*)malloc(sizeof(Book));
newNode->title = strcpy(newNode->title, title);
newNode->author = strcpy(newNode->author, author);
newNode->price = price;
newNode->next = *head;
*head = newNode;
}
```
2. **读入图书信息**:
由于这是在内存中操作,实际读取需要从用户输入或其他数据源获取,这里简化为直接赋值:
```c
void input(Book** head) {
// 这里仅做假设,实际应有输入处理
char title[50], author[50];
float price;
scanf("Title: %s Author: %s Price: %f", title, author, &price);
insert(head, title, author, price);
}
```
3. **计算平均价格**:
```c
float averagePrice(Book* head) {
if (head == NULL) return 0.0;
float sum = 0.0, count = 1.0;
Book* temp = head;
while (temp != NULL) {
sum += temp->price;
count++;
temp = temp->next;
}
return sum / count;
}
```
4. **查找价格最高图书**:
```c
Book* findHighestPrice(Book* head) {
if (head == NULL) return NULL;
Book* maxPriceNode = head;
Book* temp = head->next;
while (temp != NULL) {
if (temp->price > maxPriceNode->price) {
maxPriceNode = temp;
}
temp = temp->next;
}
return maxPriceNode;
}
```
5. **插入新图书**:
```c
void insertAtPosition(Book** head, int position, char* title, char* author, float price) {
if (position <= 0) {
insert(head, title, author, price);
} else {
Book* newNode = (Book*)malloc(sizeof(Book));
newNode->title = strcpy(newNode->title, title);
newNode->author = strcpy(newNode->author, author);
newNode->price = price;
Book* prev = *head;
for (int i = 1; i < position - 1 && prev != NULL; ++i) {
prev = prev->next;
}
if (prev == NULL) {
printf("Invalid position!\n");
return;
}
newNode->next = prev->next;
prev->next = newNode;
}
}
```
6. **删除指定位置的图书**:
```c
void deleteAtPosition(Book** head, int position) {
if (position <= 0 || *head == NULL) {
printf("Invalid position!\n");
return;
}
if (position == 1) {
Book* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Book* curr = *head;
for (int i = 1; i < position - 1 && curr != NULL; ++i) {
curr = curr->next;
}
if (curr == NULL) {
printf("Invalid position!\n");
return;
}
Book* nextNode = curr->next;
curr->next = nextNode->next;
free(nextNode);
}
```
记得在程序结束时释放所有的链表节点:
```c
void cleanup(Book* head) {
Book* temp = head;
while (temp != NULL) {
Book* nextTemp = temp->next;
free(temp);
temp = nextTemp;
}
}
```
阅读全文
相关推荐
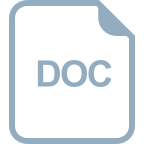
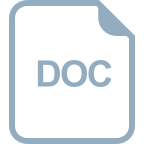
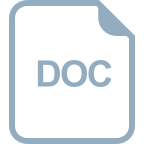


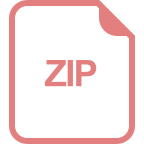
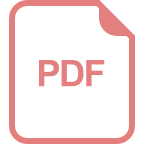
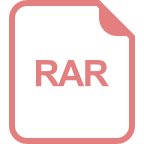
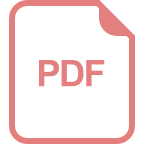
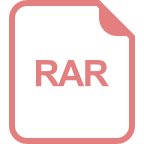
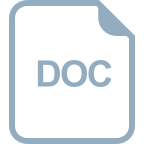

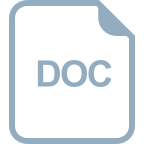
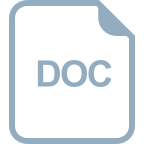
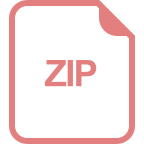