用python写一段三点坐标计算角度的代码
时间: 2023-05-14 17:04:13 浏览: 43
以下是用 Python 编写的计算三点坐标角度的代码:
```python
import math
def calculate_angle(x1, y1, x2, y2, x3, y3):
# 计算向量
ux1 = x1 - x2
uy1 = y1 - y2
ux2 = x3 - x2
uy2 = y3 - y2
# 计算向量长度
len1 = math.sqrt(ux1 ** 2 + uy1 ** 2)
len2 = math.sqrt(ux2 ** 2 + uy2 ** 2)
# 计算向量点积
dot = ux1 * ux2 + uy1 * uy2
# 计算余弦值
cos_value = dot / (len1 * len2)
# 计算角度并转换为度数
angle = math.acos(cos_value) * 180 / math.pi
return angle
# 示例
angle = calculate_angle(0, 0, 1, 0, 1, 1)
print(angle)
```
该代码接受三个点的坐标作为参数,返回第二个点所在的角度。例如,上面的示例计算了三个点 (0, 0), (1, 0), (1, 1) 所组成的角度,结果为 45 度。
相关问题
用python写一段三点坐标计算密位角的代码
以下是用 Python 编写的计算密位角的代码:
```python
import math
def calculate_bearing(lat1, lon1, lat2, lon2):
"""
Calculate the bearing between two points on Earth.
"""
# Convert latitude and longitude to radians
lat1 = math.radians(lat1)
lon1 = math.radians(lon1)
lat2 = math.radians(lat2)
lon2 = math.radians(lon2)
# Calculate the difference between the longitudes
delta_lon = lon2 - lon1
# Calculate the bearing
y = math.sin(delta_lon) * math.cos(lat2)
x = math.cos(lat1) * math.sin(lat2) - math.sin(lat1) * math.cos(lat2) * math.cos(delta_lon)
bearing = math.atan2(y, x)
# Convert bearing to degrees
bearing = math.degrees(bearing)
# Normalize bearing to range 0-360
bearing = (bearing + 360) % 360
return bearing
```
这个函数接受四个参数:起点纬度、起点经度、终点纬度和终点经度。它返回两个点之间的方位角,以度为单位。
写段python代码,根据三点坐标计算角度
Sure, here's the Python code to calculate the angle between three points:
```
import math
def calculate_angle(x1, y1, x2, y2, x3, y3):
# calculate distances between the points
a = math.sqrt((x2-x1)**2 + (y2-y1)**2)
b = math.sqrt((x3-x2)**2 + (y3-y2)**2)
c = math.sqrt((x3-x1)**2 + (y3-y1)**2)
# calculate angle using law of cosines
angle_in_radians = math.acos((a**2 + b**2 - c**2) / (2 * a * b))
# convert angle from radians to degrees
angle_in_degrees = math.degrees(angle_in_radians)
return angle_in_degrees
# example usage
x1, y1 = 0, 0
x2, y2 = 1, 1
x3, y3 = 2, 0
angle = calculate_angle(x1, y1, x2, y2, x3, y3)
print(f"The angle between ({x1}, {y1}), ({x2}, {y2}), and ({x3}, {y3}) is {angle} degrees.")
```
Hope this helps! Let me know if you have any more questions.
相关推荐
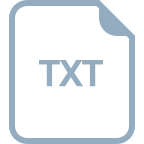
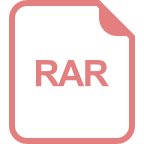
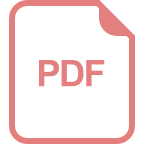












