使用beautifulsoup获得picture标签下的img标签的图片url
时间: 2024-05-04 12:22:17 浏览: 9
假设我们有以下的HTML代码:
```
<picture>
<source srcset="image.webp" type="image/webp">
<img src="image.jpg" alt="An image">
</picture>
```
可以使用BeautifulSoup库来获取`<picture>`标签下的`<img>`标签的图片URL,代码如下:
```python
from bs4 import BeautifulSoup
html = """
<picture>
<source srcset="image.webp" type="image/webp">
<img src="image.jpg" alt="An image">
</picture>
"""
soup = BeautifulSoup(html, 'html.parser')
img_tag = soup.picture.img
img_url = img_tag['src']
print(img_url) # 输出:image.jpg
```
此处我们首先将HTML代码传入BeautifulSoup库中,然后使用`soup.picture.img`获取`<picture>`标签下的`<img>`标签,最后获取`<img>`标签的`src`属性即可得到图片的URL。
相关问题
用BeautifulSoup解析指定标签下的href
可以使用BeautifulSoup的`find_all`方法来查找指定标签下的所有子标签,然后再通过子标签的属性来获取href。
下面是一个示例代码:
```python
from bs4 import BeautifulSoup
html = '''
<html>
<body>
<div class="links">
<a href="https://www.google.com">Google</a>
<a href="https://www.baidu.com">Baidu</a>
<a href="https://www.yahoo.com">Yahoo</a>
</div>
</body>
</html>
'''
soup = BeautifulSoup(html, 'html.parser')
# 查找div标签下的所有a标签
links = soup.find('div', {'class': 'links'}).find_all('a')
# 输出所有a标签的href属性值
for link in links:
print(link['href'])
```
输出结果:
```
https://www.google.com
https://www.baidu.com
https://www.yahoo.com
```
在上面的代码中,我们首先使用`find`方法查找`class`属性为`links`的`div`标签,然后使用`find_all`方法查找该标签下的所有`a`标签。最后,我们使用`link['href']`来获取每个`a`标签的`href`属性值。
python用beautifulsoup爬取div标签下的内容
非常好的问题,可以使用BeautifulSoup来实现这个目标。首先需要安装bs4库,然后可以使用以下代码来实现:
```
from bs4 import BeautifulSoup
import requests
url = 'YOUR_URL_HERE'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
div_contents = soup.find('div', {'class': 'YOUR_CLASS_HERE'}).contents
for content in div_contents:
print(content)
```
其中,`YOUR_URL_HERE` 替换成需要爬取的网页地址,`YOUR_CLASS_HERE` 替换成需要爬取的 div 标签的 class 名称。这段代码会将对应 div 标签下的所有内容打印出来。
相关推荐
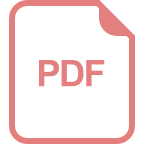
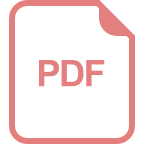
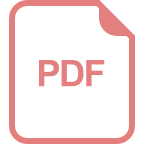












