实现一个分类(贝叶斯)算法实例
时间: 2024-04-29 13:22:25 浏览: 86
以下是一个简单的贝叶斯分类算法的示例代码,用于将文本分类为“垃圾邮件”或“非垃圾邮件”:
```python
import re
import math
class SpamClassifier:
def __init__(self):
self.spam_words = {}
self.ham_words = {}
self.spam_count = 0
self.ham_count = 0
self.total_count = 0
def train(self, texts, labels):
for i in range(len(texts)):
words = re.findall(r'\w+', texts[i].lower())
if labels[i] == 'spam':
self.spam_count += 1
for word in words:
self.spam_words[word] = self.spam_words.get(word, 0) + 1
self.total_count += 1
else:
self.ham_count += 1
for word in words:
self.ham_words[word] = self.ham_words.get(word, 0) + 1
self.total_count += 1
def predict(self, text):
words = re.findall(r'\w+', text.lower())
spam_prob = math.log(self.spam_count / (self.spam_count + self.ham_count))
ham_prob = math.log(self.ham_count / (self.spam_count + self.ham_count))
for word in words:
if word in self.spam_words:
spam_prob += math.log((self.spam_words[word] + 1) / (self.total_count + len(self.spam_words)))
else:
spam_prob += math.log(1 / (self.total_count + len(self.spam_words)))
if word in self.ham_words:
ham_prob += math.log((self.ham_words[word] + 1) / (self.total_count + len(self.ham_words)))
else:
ham_prob += math.log(1 / (self.total_count + len(self.ham_words)))
if spam_prob > ham_prob:
return 'spam'
else:
return 'ham'
```
在这个示例中,我们使用了一个简单的贝叶斯分类算法来对文本进行分类。首先,我们使用训练数据来计算垃圾邮件和非垃圾邮件中每个单词出现的次数,并计算每个类别的文本总数。然后,对于新的文本,我们计算每个类别的概率,并根据概率大小进行分类。
在训练过程中,我们使用了Python的正则表达式模块re来提取文本中的单词。我们还使用了Python的字典来存储每个单词在垃圾邮件和非垃圾邮件中出现的次数。
在预测过程中,我们使用了Python的math库来计算对数概率,以避免数值下溢。我们还使用了Python的if语句来根据概率大小进行分类,并返回相应的标签。
需要注意的是,这个示例并不完整,可能无法处理所有情况。在实际应用中,需要根据具体情况进行修改和优化。
阅读全文
相关推荐
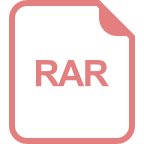
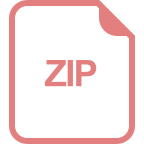
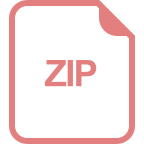
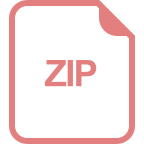
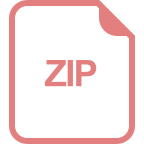
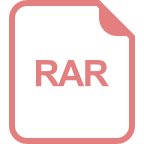
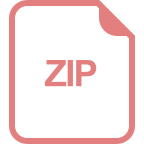
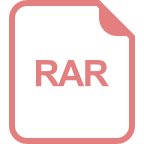
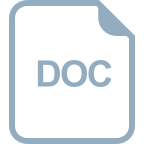
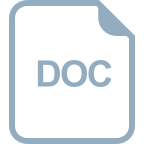
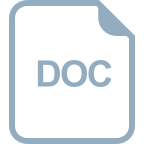
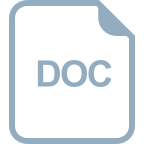
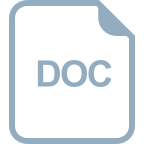
