砂轮片是像光碟一样的圆形,中间镂空的,拍照时,由于不是正对摄像机放置,导致拍到的图像,砂轮片看起来是椭圆,用什么进行图像处理比较好,请给我一个c++完整代码
时间: 2024-06-08 21:12:15 浏览: 129
对于这个问题,可以使用OpenCV库进行图像处理。处理步骤如下:
1. 读取图像,并将其转换为灰度图像。
2. 使用Canny边缘检测算法检测砂轮片的边缘。
3. 使用霍夫圆变换算法检测出砂轮片的圆形轮廓。
4. 根据检测出来的圆形轮廓,计算出砂轮片的旋转角度,并进行旋转矫正。
5. 将矫正后的图像保存。
以下是完整的C++代码示例:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main()
{
Mat image = imread("sand_wheel.jpg", IMREAD_GRAYSCALE); // 读取图像并转换为灰度图像
Mat edges; // 存储边缘图像
Mat circles; // 存储检测出来的圆形轮廓
// Canny边缘检测
Canny(image, edges, 50, 200);
// 霍夫圆变换
HoughCircles(edges, circles, HOUGH_GRADIENT, 1, image.rows / 16, 100, 30, 0, 0);
// 计算砂轮片的旋转角度
int angle = 0;
if (circles.rows == 1)
{
Point center(cvRound(circles.at<float>(0, 0)), cvRound(circles.at<float>(0, 1)));
int radius = cvRound(circles.at<float>(0, 2));
Mat rotated;
getRotationMatrix2D(center, 0, 1.0);
warpAffine(image, rotated, getRotationMatrix2D(center, -90, 1.0), image.size());
Mat crop = rotated(Rect(center.x - radius, center.y - radius, radius * 2, radius * 2));
Mat straightened;
getRotationMatrix2D(center, angle, 1.0);
warpAffine(crop, straightened, getRotationMatrix2D(center, angle, 1.0), crop.size());
imshow("Straightened", straightened);
}
else
{
cout << "Circle detection failed!" << endl;
}
waitKey(0); // 等待用户按键
return 0;
}
```
在这个例子中,我们使用了OpenCV的Canny边缘检测算法和霍夫圆变换算法来检测砂轮片的圆形轮廓。然后,我们使用旋转矩阵来计算出砂轮片的旋转角度,并进行旋转矫正。最后,我们显示矫正后的图像。
需要注意的是,这个例子中只考虑了检测到一个圆形轮廓的情况,如果有多个圆形轮廓,需要进行额外的处理。
阅读全文
相关推荐
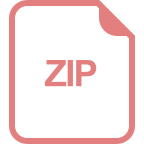

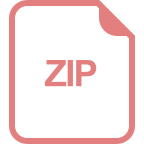
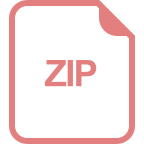
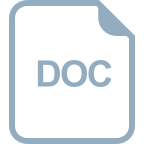
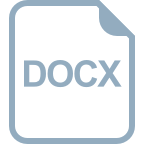
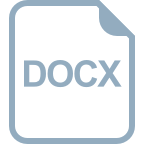
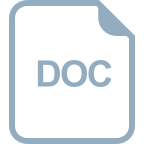
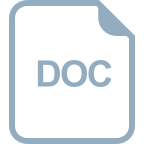
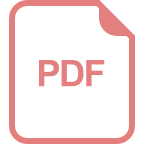
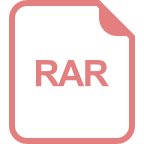
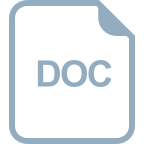
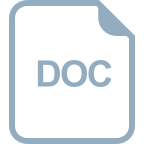