python实现softmax分类平面上的n类,每类m个二维数据点并可视化
时间: 2024-05-07 15:16:26 浏览: 10
可以使用Python的NumPy和Matplotlib库来实现。首先,需要生成n类m个二维数据点,并为每个数据点分配一个标签。然后,使用softmax函数将每个数据点的标签转换为概率分布。最后,使用Matplotlib将数据点可视化在平面上。
以下是一个示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成数据
n = 3 # 类别数
m = 100 # 每类数据点数
data = np.zeros((n*m, 2))
labels = np.zeros(n*m, dtype=int)
for i in range(n):
theta = np.linspace(i*2*np.pi/n, (i+1)*2*np.pi/n, m)
radius = np.random.normal(0.5, 0.1, m)
x = radius * np.cos(theta) + np.random.normal(0, 0.1, m)
y = radius * np.sin(theta) + np.random.normal(0, 0.1, m)
data[i*m:(i+1)*m, :] = np.stack((x, y), axis=1)
labels[i*m:(i+1)*m] = i
# 可视化数据
plt.scatter(data[:, 0], data[:, 1], c=labels, cmap='viridis')
plt.show()
# 计算softmax概率分布
def softmax(z):
exp_z = np.exp(z - np.max(z, axis=1, keepdims=True))
return exp_z / np.sum(exp_z, axis=1, keepdims=True)
# 计算每个数据点的概率分布
scores = np.dot(data, np.random.randn(2, n)) # 使用随机权重矩阵
probs = softmax(scores)
# 可视化概率分布
plt.scatter(data[:, 0], data[:, 1], c=probs, cmap='viridis')
plt.show()
```
这段代码首先使用高斯分布生成n类m个二维数据点,然后使用Matplotlib将数据点可视化在平面上。接着,使用softmax函数将每个数据点的标签转换为概率分布,并再次使用Matplotlib将概率分布可视化在平面上。注意,权重矩阵是随机初始化的,所以每次运行结果可能会有所不同。
相关推荐
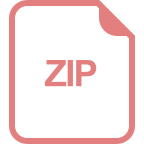









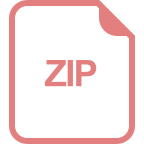
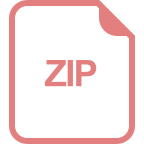
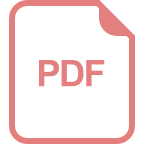