Tensorflow 实现自定义层:改写常规模型中的任意模块或者层,并实现对已下载好的mnist数据集进行图像分类,结果可视化,具体代码
时间: 2023-12-03 13:44:46 浏览: 34
首先,我们需要导入所需的库和数据集:
```python
import tensorflow as tf
import matplotlib.pyplot as plt
# 加载MNIST数据集
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
```
接下来,我们可以定义一个自定义层,此处以一个简单的全连接层为例:
```python
class MyDenseLayer(tf.keras.layers.Layer):
def __init__(self, units=32, input_dim=784):
super(MyDenseLayer, self).__init__()
self.w = self.add_weight(shape=(input_dim, units),
initializer='random_normal',
trainable=True)
self.b = self.add_weight(shape=(units,),
initializer='zeros',
trainable=True)
def call(self, inputs):
return tf.matmul(inputs, self.w) + self.b
```
这个自定义层包含一个权重矩阵和一个偏置向量,输入数据将通过这两个参数进行线性变换。我们可以使用 `add_weight` 方法来创建可训练的权重和偏置。
接下来,我们可以构建包含自定义层的模型:
```python
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
MyDenseLayer(units=128),
tf.keras.layers.Dense(units=10, activation='softmax')
])
```
这个模型包含一个展平层,一个自定义全连接层和一个输出层。展平层将输入的二维图像展平为一维向量,自定义全连接层将一维向量转换为另一个一维向量,输出层将该向量映射到10个类别的概率分布上。
接下来,我们可以编译模型并训练:
```python
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=5)
```
最后,我们可以使用测试集评估模型并可视化结果:
```python
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
predictions = model.predict(test_images)
# 可视化结果
plt.figure(figsize=(10, 10))
for i in range(25):
plt.subplot(5, 5, i+1)
plt.xticks([])
plt.yticks([])
plt.grid(False)
plt.imshow(test_images[i], cmap=plt.cm.binary)
predicted_label = tf.argmax(predictions[i])
true_label = test_labels[i]
if predicted_label == true_label:
color = 'green'
else:
color = 'red'
plt.xlabel("{} ({})".format(predicted_label,
true_label),
color=color)
plt.show()
```
这段代码将显示测试集中的前25个图像,并用绿色或红色标记模型的预测结果是否与真实标签匹配。
完整的代码如下:
```python
import tensorflow as tf
import matplotlib.pyplot as plt
# 加载MNIST数据集
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
class MyDenseLayer(tf.keras.layers.Layer):
def __init__(self, units=32, input_dim=784):
super(MyDenseLayer, self).__init__()
self.w = self.add_weight(shape=(input_dim, units),
initializer='random_normal',
trainable=True)
self.b = self.add_weight(shape=(units,),
initializer='zeros',
trainable=True)
def call(self, inputs):
return tf.matmul(inputs, self.w) + self.b
# 构建模型
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
MyDenseLayer(units=128),
tf.keras.layers.Dense(units=10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=5)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
# 预测结果并可视化
predictions = model.predict(test_images)
plt.figure(figsize=(10, 10))
for i in range(25):
plt.subplot(5, 5, i+1)
plt.xticks([])
plt.yticks([])
plt.grid(False)
plt.imshow(test_images[i], cmap=plt.cm.binary)
predicted_label = tf.argmax(predictions[i])
true_label = test_labels[i]
if predicted_label == true_label:
color = 'green'
else:
color = 'red'
plt.xlabel("{} ({})".format(predicted_label,
true_label),
color=color)
plt.show()
```
相关推荐
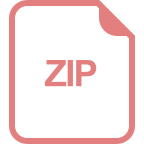
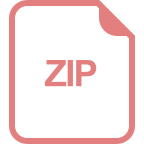















