编写一个程序,采用sklearn中的LogisticRegression逻辑回归模型对iris数据集进行多分类,并使数据可视化
时间: 2024-02-20 14:57:54 浏览: 23
好的,以下是一个可以对iris数据集进行多分类并可视化的Python程序:
```python
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 加载iris数据集
iris = load_iris()
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2, random_state=42)
# 创建逻辑回归模型
model = LogisticRegression(multi_class='multinomial', solver='lbfgs')
# 在训练集上拟合模型
model.fit(X_train, y_train)
# 在测试集上评估模型精度
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("模型精度为:", accuracy)
# 可视化数据集和模型预测结果
plt.figure(figsize=(10, 6))
# 绘制数据集
plt.scatter(X_test[:, 0], X_test[:, 1], c=y_test)
# 绘制模型预测结果
x_min, x_max = X_test[:, 0].min() - 0.5, X_test[:, 0].max() + 0.5
y_min, y_max = X_test[:, 1].min() - 0.5, X_test[:, 1].max() + 0.5
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.02), np.arange(y_min, y_max, 0.02))
Z = model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.3)
plt.xlabel('Sepal length')
plt.ylabel('Sepal width')
plt.title('Logistic Regression for Iris Classification')
plt.show()
```
这个程序与之前的示例非常相似,不同之处在于我们使用Matplotlib库将数据集和模型的预测结果可视化。我们使用plt.scatter函数绘制数据集,使用np.meshgrid函数生成网格点,使用model.predict函数获取模型的预测结果,并使用plt.contourf函数绘制预测结果的等高线。最后,我们添加了一些标签和标题,并使用plt.show函数显示图形。
相关推荐
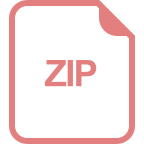
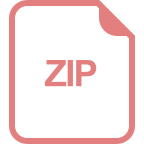
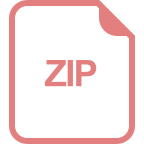











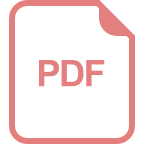
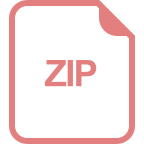
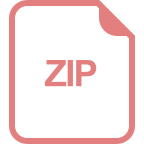