网页抓取(天气温度数据),将抓取的数据进行处理,结果保存进入mysql,利用pyecharts处理结果可视化,flask联通前后端,(mysql到pyecharts网页),有首页界面(bootstrap),有登录界面,这个实践项目在vscode上的代码示例教程
时间: 2024-06-08 09:06:28 浏览: 223
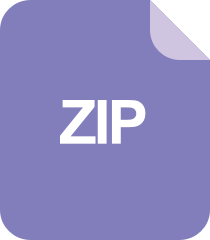
Python+Flask爬虫数据可视化分析大作业(说明文档+数据库文件)高分项目
这个实践项目需要分为多个步骤来完成,下面是一些大致的步骤:
1. 确定项目需求和目标:这个项目的目标是抓取天气温度数据,并将处理结果保存到mysql数据库中。然后,使用pyecharts将数据可视化,并通过flask构建前后端网页,实现mysql到pyecharts的数据传递和展示。
2. 安装必要的库:在vscode上打开终端,安装必要的库,如requests、beautifulsoup4、pymysql、pyecharts等。可以使用以下命令来安装:
```
pip install requests beautifulsoup4 pymysql pyecharts flask
```
3. 编写抓取天气温度数据的Python脚本:创建一个Python文件,使用requests和beautifulsoup4库抓取天气温度数据,并将数据进行处理,最后将结果保存到mysql数据库中。可以使用以下代码作为参考:
```python
import requests
from bs4 import BeautifulSoup
import pymysql
# 获取天气温度数据
def get_weather_data():
url = 'http://www.weather.com.cn/weather/101010100.shtml'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
temperature = soup.select('.tem')[0].text.strip()
weather = soup.select('.wea')[0].text.strip()
return temperature, weather
# 将数据保存到mysql数据库中
def save_to_mysql(temperature, weather):
connection = pymysql.connect(
host='localhost',
user='root',
password='password',
database='weather_db'
)
cursor = connection.cursor()
sql = "INSERT INTO weather (temperature, weather) VALUES (%s, %s)"
cursor.execute(sql, (temperature, weather))
connection.commit()
connection.close()
if __name__ == '__main__':
temperature, weather = get_weather_data()
save_to_mysql(temperature, weather)
```
4. 创建一个MySQL数据库:在MySQL数据库中创建一个名为weather_db的数据库,并创建一个名为weather的表。可以使用以下命令来创建:
```sql
CREATE DATABASE weather_db;
USE weather_db;
CREATE TABLE weather (
id INT(11) NOT NULL AUTO_INCREMENT,
temperature VARCHAR(10) NOT NULL,
weather VARCHAR(20) NOT NULL,
PRIMARY KEY (id)
);
```
5. 编写pyecharts可视化脚本:创建一个Python文件,使用pymysql库从mysql数据库中获取数据,并使用pyecharts库将数据可视化。可以使用以下代码作为参考:
```python
import pymysql
from pyecharts.charts import Line
from pyecharts import options as opts
# 从mysql数据库中获取数据
def get_data_from_mysql():
connection = pymysql.connect(
host='localhost',
user='root',
password='password',
database='weather_db'
)
cursor = connection.cursor()
sql = "SELECT * FROM weather"
cursor.execute(sql)
result = cursor.fetchall()
connection.close()
return result
# 将数据可视化
def render_chart(data):
x_data = [i[0] for i in data]
y_data = [i[1] for i in data]
chart = Line()
chart.add_xaxis(x_data)
chart.add_yaxis('温度', y_data)
chart.set_global_opts(title_opts=opts.TitleOpts(title='天气温度'))
return chart
if __name__ == '__main__':
data = get_data_from_mysql()
chart = render_chart(data)
chart.render('render.html')
```
6. 编写flask应用程序:创建一个Python文件,使用flask构建前后端网页,并将pyecharts生成的HTML文件嵌入到网页中,实现mysql到pyecharts的数据传递和展示。可以使用以下代码作为参考:
```python
from flask import Flask, render_template, request
import pymysql
app = Flask(__name__)
# 从mysql数据库中获取数据
def get_data_from_mysql():
connection = pymysql.connect(
host='localhost',
user='root',
password='password',
database='weather_db'
)
cursor = connection.cursor()
sql = "SELECT * FROM weather"
cursor.execute(sql)
result = cursor.fetchall()
connection.close()
return result
# 渲染flask模板
@app.route('/')
def index():
data = get_data_from_mysql()
return render_template('index.html', data=data)
if __name__ == '__main__':
app.run(debug=True)
```
7. 创建flask模板和静态文件:在项目目录下创建一个名为templates的文件夹,创建一个名为index.html的模板文件,使用pyecharts生成的HTML文件嵌入到模板中,实现数据可视化。在项目目录下创建一个名为static的文件夹,将Bootstrap的CSS和JS文件放入其中。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>天气温度</title>
<link href="{{ url_for('static', filename='css/bootstrap.min.css') }}" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="text-center">天气温度</h1>
<div class="row">
<div class="col-md-6">
<table class="table table-bordered">
<thead>
<tr>
<th>日期</th>
<th>温度</th>
<th>天气</th>
</tr>
</thead>
<tbody>
{% for item in data %}
<tr>
<td>{{ item[0] }}</td>
<td>{{ item[1] }}</td>
<td>{{ item[2] }}</td>
</tr>
{% endfor %}
</tbody>
</table>
</div>
<div class="col-md-6">
<div id="chart"></div>
</div>
</div>
</div>
<script src="{{ url_for('static', filename='js/bootstrap.min.js') }}"></script>
<script src="{{ url_for('static', filename='js/jquery-3.5.1.min.js') }}"></script>
<script src="{{ url_for('static', filename='js/echarts.min.js') }}"></script>
<script>
var chart = echarts.init(document.getElementById('chart'));
chart.setOption({
tooltip: {
trigger: 'axis'
},
legend: {
data: ['温度']
},
xAxis: {
type: 'category',
data: [{% for item in data %}"{{ item[0] }}",{% endfor %}]
},
yAxis: {
type: 'value'
},
series: [{
name: '温度',
type: 'line',
data: [{% for item in data %}{{ item[1] }}, {% endfor %}]
}]
});
</script>
</body>
</html>
```
8. 运行flask应用程序:在vscode中打开终端,运行flask应用程序,然后在浏览器中访问http://127.0.0.1:5000/,即可看到数据可视化的结果。
以上就是这个实践项目的大致步骤,具体实现过程中可能需要根据实际情况进行一些调整。
阅读全文
相关推荐
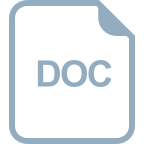
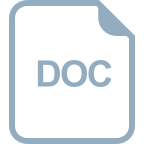







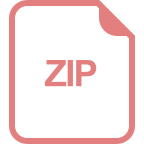
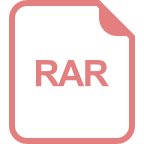
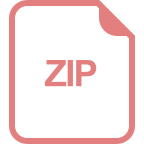
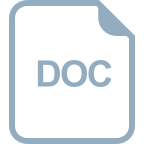
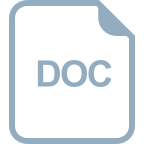

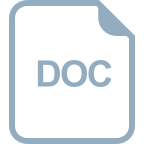
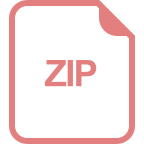