调用api函数发送请求,若5秒后未请求成功,则调用warning函数发出警告。其中,api函数在请求成功的情况下会返回一个promise使用javascript编程实现
时间: 2024-06-10 21:06:45 浏览: 141
Sure, here is a possible implementation using JavaScript:
```
function sendRequestWithTimeout(apiFunction, timeout) {
let timeoutId;
const promise = new Promise((resolve, reject) => {
// Set timeout
timeoutId = setTimeout(() => {
reject('Request timed out');
}, timeout);
// Call API function
apiFunction().then((response) => {
resolve(response);
}).catch((error) => {
reject(error);
});
});
// Cancel timeout when request is fulfilled
promise.finally(() => clearTimeout(timeoutId));
return promise;
}
function apiFunction() {
// Call API function here and return a Promise
}
function warning() {
// Show warning message or take any other action
}
sendRequestWithTimeout(apiFunction, 5000).then((response) => {
// Handle successful response here
}).catch((error) => {
if (error === 'Request timed out') {
warning();
} else {
// Handle other errors
}
});
```
In the above code, `sendRequestWithTimeout` is a wrapper function that takes an `apiFunction` and a `timeout` in milliseconds. It returns a Promise that resolves with the response if the `apiFunction` is successful within the timeout period, or rejects with an error message if the `apiFunction` fails or takes longer than the timeout period. The timeout is implemented using `setTimeout`, and is cancelled when the Promise is fulfilled (whether resolved or rejected).
You can use this function to wrap any API function that returns a Promise, like so:
```
sendRequestWithTimeout(() => fetch('https://example.com/api/data'), 5000)
```
Note that this code doesn't make any requests or handle any warnings directly, it only provides a reusable function to wrap API calls with a timeout. You'll need to adapt it to your specific use case by replacing `apiFunction` and `warning` with your own implementations.
阅读全文
相关推荐
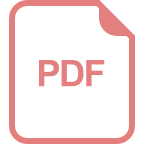
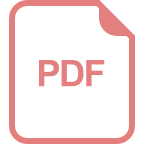
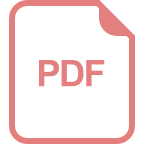















