使用java代码编写测试单点登录
时间: 2023-08-01 17:08:33 浏览: 107
要测试单点登录(SSO)的实现,你可以使用Java编写单元测试来验证登录功能是否正常工作。以下是一个简单的示例:
1. 首先,确保你的应用程序已经实现了单点登录功能,并且你已经有一个可以进行登录的页面。
2. 创建一个测试类,例如`SSOTest`,并在该类上添加`@RunWith(SpringRunner.class)`和`@SpringBootTest`注解,以便使用Spring Boot进行集成测试。
```java
import org.junit.Assert;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.web.client.RestTemplate;
@RunWith(SpringRunner.class)
@SpringBootTest
public class SSOTest {
@Autowired
private RestTemplate restTemplate;
@Test
public void testLogin() {
String username = "your_username";
String password = "your_password";
// 构建登录请求
String loginUrl = "http://localhost:8080/login"; // 替换为你的登录页面URL
String requestBody = "username=" + username + "&password=" + password;
ResponseEntity<String> response = restTemplate.postForEntity(loginUrl, requestBody, String.class);
// 验证登录结果
Assert.assertEquals(HttpStatus.OK, response.getStatusCode());
// 如果登录成功后,可以获取响应中的Cookie或其他标识来验证登录状态
// ...
}
}
```
3. 在测试方法中,你可以使用`RestTemplate`执行登录请求,并验证返回的响应状态码和登录结果。
4. 运行测试类,你应该能够看到测试结果。如果登录成功,响应状态码应该是200 OK,并且可以根据实际情况验证登录状态。
请注意,这只是一个简单的示例,实际的测试可能会更加复杂,具体取决于你的应用程序的实现方式和需求。你可以根据自己的情况进行适当调整和扩展。另外,还可以使用其他测试工具和框架(如JUnit、MockMvc等)来进行更全面的测试。
阅读全文
相关推荐
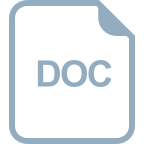
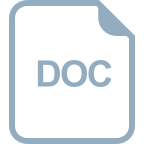
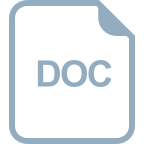
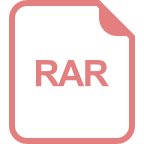
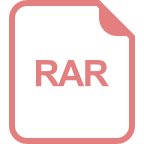
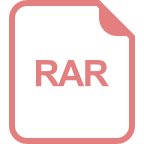
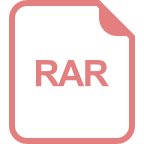
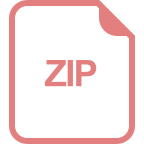
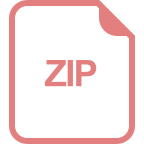
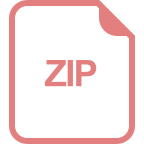
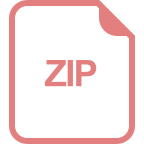
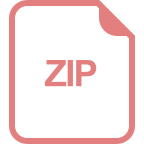
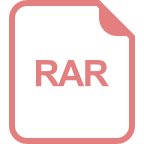
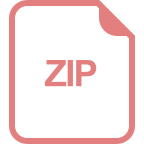
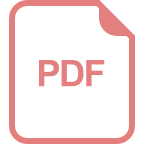
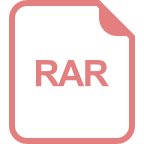
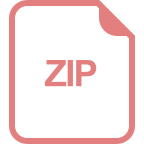
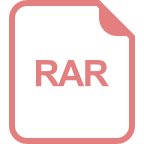
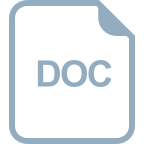