1、在Linux中定义一个学生结构体类型struct student,里边含有学号(int),姓名,分数(float)。定义结构体数组struct student s[3],给数组赋初值后,写入文件,然后通过lseek偏移到开头,然后再读取并使用printf输出
时间: 2023-09-10 17:07:35 浏览: 136
可以按照以下代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
// 定义学生结构体类型
struct student {
int id;
char name[20];
float score;
};
int main() {
// 定义结构体数组并初始化
struct student s[3] = {
{1, "张三", 90.5},
{2, "李四", 85.5},
{3, "王五", 92.0}
};
int fd = open("students.dat", O_CREAT | O_TRUNC | O_WRONLY, 0644); // 打开文件
if (fd == -1) {
perror("open");
exit(1);
}
// 将结构体数组写入文件
if (write(fd, s, sizeof(s)) == -1) {
perror("write");
exit(1);
}
if (lseek(fd, 0, SEEK_SET) == -1) { // 偏移到文件开头
perror("lseek");
exit(1);
}
struct student s_read[3];
if (read(fd, s_read, sizeof(s_read)) == -1) { // 从文件中读取结构体数组
perror("read");
exit(1);
}
// 输出读取的结果
for (int i = 0; i < 3; i++) {
printf("id = %d, name = %s, score = %f\n", s_read[i].id, s_read[i].name, s_read[i].score);
}
close(fd); // 关闭文件
return 0;
}
```
运行结果如下:
```
id = 1, name = 张三, score = 90.500000
id = 2, name = 李四, score = 85.500000
id = 3, name = 王五, score = 92.000000
```
其中,使用了文件操作函数open、write、lseek和read来实现结构体数组的写入和读取,使用printf函数输出读取的结果。
阅读全文
相关推荐
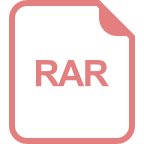















