Rosen梯度投影法 matlab代码
时间: 2023-10-04 12:13:48 浏览: 220
Rosen梯度投影法是一种非线性规划优化方法,可用于求解约束条件下的最优解。以下是该算法的MATLAB实现代码:
```matlab
function [x, fval] = rosen_gradient_projection(f, x0, A, b, lb, ub, maxiter, tol)
% ROSEN_GRADIENT_PROJECTION - Rosen's gradient projection algorithm for nonlinear programming
% [X, FVAL] = ROSEN_GRADIENT_PROJECTION(F, X0, A, B, LB, UB, MAXITER, TOL) finds the minimum of
% a nonlinear constrained function F, using the Rosen's gradient projection algorithm. X0 is the
% initial guess, A and B are the linear inequality constraints, LB and UB are the lower and upper
% bounds of the variables, MAXITER is the maximum number of iterations, and TOL is the tolerance
% for the stopping criterion. The function returns the minimum X and the function value FVAL at X.
% Set default values if not specified
if nargin < 8 || isempty(tol)
tol = 1e-6;
end
if nargin < 7 || isempty(maxiter)
maxiter = 1000;
end
if nargin < 6 || isempty(ub)
ub = inf(size(x0));
end
if nargin < 5 || isempty(lb)
lb = -inf(size(x0));
end
if nargin < 4 || isempty(b)
b = [];
end
if nargin < 3 || isempty(A)
A = [];
end
% Initialize variables
n = length(x0);
x = x0;
fval = f(x);
g = gradient(f, x);
k = 0;
% Main loop
while k < maxiter && norm(g, inf) > tol
% Compute the projected gradient
gproj = project_gradient(x, g, A, b, lb, ub);
% Compute the step size
alpha = linesearch(f, x, gproj);
% Update x and g
x = x - alpha * gproj;
fval = f(x);
g = gradient(f, x);
% Increment iteration counter
k = k + 1;
end
% Display warning if maximum number of iterations reached
if k == maxiter
warning('Maximum number of iterations reached.');
end
end
function gproj = project_gradient(x, g, A, b, lb, ub)
% PROJECT_GRADIENT - Project the gradient onto the feasible region
% GPROJ = PROJECT_GRADIENT(X, G, A, B, LB, UB) projects the gradient G onto the feasible region
% defined by the linear inequality constraints A*X <= B and the lower and upper bounds LB and UB.
% Compute the projection of the gradient onto the feasible region
gproj = g;
if ~isempty(A)
gproj = gproj - A' * ((A * x - b) ./ sum(A.^2, 2))';
end
gproj(x == lb & gproj < 0) = 0;
gproj(x == ub & gproj > 0) = 0;
end
function alpha = linesearch(f, x, g)
% LINESEARCH - Compute the step size using line search
% ALPHA = LINESEARCH(F, X, G) computes the step size ALPHA using the line search algorithm.
% Set initial values
alpha = 1;
c = 1e-4;
rho = 0.9;
f0 = f(x);
g0 = g' * g;
% Main loop
while f(x - alpha * g) > f0 - c * alpha * g0
alpha = rho * alpha;
end
end
function g = gradient(f, x)
% GRADIENT - Compute the gradient of a function
% G = GRADIENT(F, X) computes the gradient G of a function F at a point X.
% Set step size
h = 1e-6;
% Initialize gradient vector
g = zeros(size(x));
% Compute gradient for each variable
for i = 1:length(x)
x1 = x;
x1(i) = x(i) + h;
x2 = x;
x2(i) = x(i) - h;
g(i) = (f(x1) - f(x2)) / (2 * h);
end
end
```
该代码实现了Rosen梯度投影法的主要步骤,并考虑了线性不等式约束和变量边界。您可以将自己的非线性约束函数作为输入参数f,并调整其他输入参数以满足您的问题需求。
相关推荐
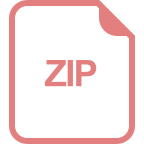
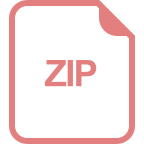














