用C或C++语言设计并实现一个可进行复数运算的演示程序。
时间: 2024-10-08 10:23:26 浏览: 28
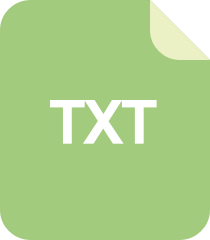
复数四则运算(利用C++的复数库实现复数的各种数学运算)

在C++中,我们可以创建一个简单的复数类(ComplexNumber),用于基本的复数加法、减法、乘法和除法操作。以下是一个基础版本的示例:
```cpp
#include <iostream>
using namespace std;
class ComplexNumber {
private:
double real; // 实部
double imag; // 虚部
public:
// 构造函数
ComplexNumber(double r = 0, double i = 0) : real(r), imag(i) {}
// 访问器和设置器
double getReal() const { return real; }
double getImaginary() const { return imag; }
void setReal(double r) { real = r; }
void setImaginary(double i) { imag = i; }
// 复数运算
ComplexNumber add(ComplexNumber other) const {
double new_real = real + other.real;
double new_imag = imag + other.imag;
return ComplexNumber(new_real, new_imag);
}
ComplexNumber subtract(ComplexNumber other) const {
double new_real = real - other.real;
double new_imag = imag - other.imag;
return ComplexNumber(new_real, new_imag);
}
ComplexNumber multiply(ComplexNumber other) const {
double new_real = real * other.real - imag * other.imag;
double new_imag = real * other.imag + imag * other.real;
return ComplexNumber(new_real, new_imag);
}
// 如果除数不是0,进行除法运算;否则抛出异常
ComplexNumber divide(ComplexNumber other) const {
if (other.getReal() == 0 && other.getImaginary() == 0)
throw runtime_error("Cannot divide by zero.");
double denominator = other.getReal() * other.getReal() + other.getImaginary() * other.getImaginary();
double new_real = (real * other.getReal() + imag * other.getImaginary()) / denominator;
double new_imag = (imag * other.getReal() - real * other.getImaginary()) / denominator;
return ComplexNumber(new_real, new_imag);
}
// 打印复数
void print() const {
cout << "(" << real << ", " << imag << ") ";
}
};
int main() {
ComplexNumber c1(2, 3);
ComplexNumber c2(4, 5);
c1.print(); cout << "+";
c2.print(); cout << " = ";
ComplexNumber sum = c1.add(c2);
sum.print();
cout << "\nSubtraction:\n";
c1.print(); cout << "-";
c2.print(); cout << " = ";
ComplexNumber sub = c1.subtract(c2);
sub.print();
cout << "\nMultiplication:\n";
c1.print(); cout << "*";
c2.print(); cout << " = ";
ComplexNumber mul = c1.multiply(c2);
mul.print();
try {
cout << "\nDivision:\n";
c1.print(); cout << "/";
c2.print(); cout << " = ";
ComplexNumber div = c1.divide(c2);
div.print();
} catch (const exception& e) {
cerr << "Error: " << e.what() << endl;
}
return 0;
}
```
在这个例子中,我们定义了一个复数类,包含构造函数、访问器、赋值器以及基本的算术运算方法。`main()`函数展示了如何创建复数对象,并进行各种运算。
阅读全文
相关推荐
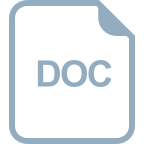
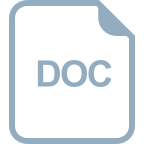

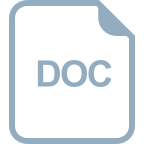
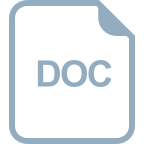
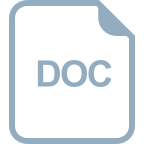
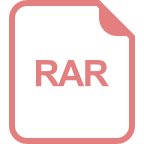
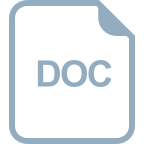
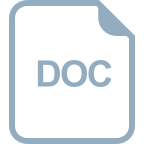
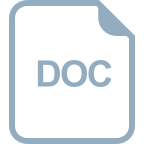
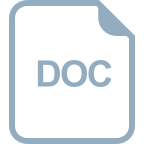
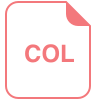




