C语言模拟停车场管理系统
时间: 2023-09-02 08:07:16 浏览: 133
停车场管理系统通常包括车辆和停车场两个主要的实体。
对于车辆,我们需要记录车牌号、入场时间、停车时长等信息。对于停车场,我们需要记录停车场的总车位数、当前空闲车位数、停车费用等信息。
以下是一个简单的 C 语言代码示例,模拟停车场管理系统:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_SIZE 100 // 停车场最大容量
// 车辆结构体
typedef struct Car {
char license[10]; // 车牌号
time_t enter_time; // 入场时间
int parked_time; // 停车时长(分钟)
} Car;
// 停车场结构体
typedef struct ParkingLot {
int total_space; // 总车位数
int available_space; // 空闲车位数
float fee_rate; // 停车费率(每小时多少元)
Car cars[MAX_SIZE]; // 停车场中的车辆数组
} ParkingLot;
// 初始化停车场
void init_parking_lot(ParkingLot *parking_lot, int total_space, float fee_rate) {
parking_lot->total_space = total_space;
parking_lot->available_space = total_space;
parking_lot->fee_rate = fee_rate;
}
// 进入停车场
void enter_parking_lot(ParkingLot *parking_lot, char *license) {
if (parking_lot->available_space <= 0) {
printf("停车场已满,无法进入!\n");
return;
}
// 生成随机入场时间
srand((unsigned int)time(NULL));
time_t enter_time = time(NULL) - rand() % 3600;
// 添加车辆到停车场
Car car = { .enter_time = enter_time, .parked_time = 0 };
strcpy(car.license, license);
parking_lot->cars[parking_lot->total_space - parking_lot->available_space] = car;
parking_lot->available_space--;
printf("车辆 %s 进入停车场,入场时间:%s", license, ctime(&enter_time));
}
// 离开停车场
void leave_parking_lot(ParkingLot *parking_lot, char *license) {
int i;
for (i = 0; i < parking_lot->total_space; i++) {
if (strcmp(parking_lot->cars[i].license, license) == 0) {
// 计算停车费用
int parked_time = (int)difftime(time(NULL), parking_lot->cars[i].enter_time) / 60;
float fee = parked_time * parking_lot->fee_rate;
printf("车辆 %s 离开停车场,停车时长:%d分钟,停车费用:%.2f元\n", license, parked_time, fee);
// 从停车场中删除车辆
int j;
for (j = i; j < parking_lot->total_space - 1; j++) {
parking_lot->cars[j] = parking_lot->cars[j + 1];
}
parking_lot->available_space++;
return;
}
}
printf("未找到车辆 %s,无法离开停车场!\n", license);
}
// 显示停车场信息
void show_parking_lot_info(ParkingLot *parking_lot) {
printf("停车场总车位数:%d,当前空闲车位数:%d,停车费率:%.2f元/小时\n", parking_lot->total_space, parking_lot->available_space, parking_lot->fee_rate);
}
int main() {
ParkingLot parking_lot;
init_parking_lot(&parking_lot, 100, 5);
enter_parking_lot(&parking_lot, "京A12345");
enter_parking_lot(&parking_lot, "京B67890");
show_parking_lot_info(&parking_lot);
leave_parking_lot(&parking_lot, "京A12345");
show_parking_lot_info(&parking_lot);
return 0;
}
```
以上代码中,我们定义了两个结构体 `Car` 和 `ParkingLot`,分别表示车辆和停车场。在 `main` 函数中,我们先初始化了一个停车场,然后模拟了两辆车进入停车场,并输出了停车场当前的信息。接着,我们让一辆车离开了停车场,并再次输出了停车场的信息。
这只是一个简单的停车场管理系统代码示例,实际的停车场管理系统还需要考虑很多其他因素,比如停车场的收费策略、车辆进出的安全性等。
阅读全文
相关推荐
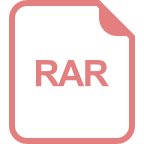
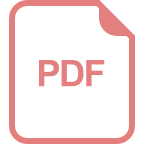
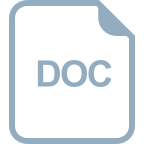
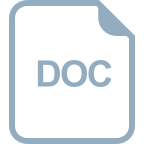
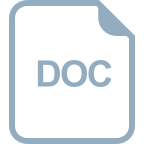
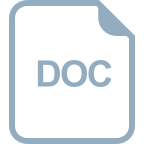
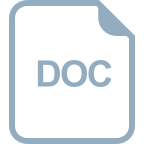
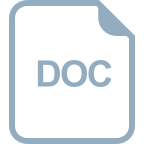
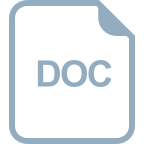
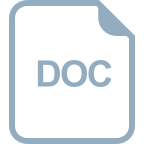
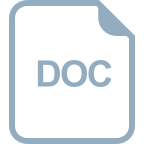
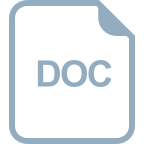
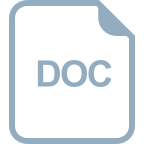
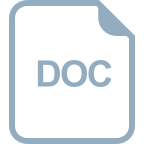
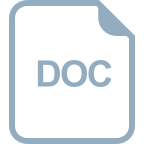
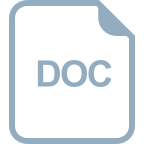
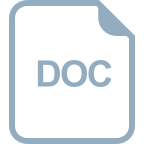