python defaultdict(array)
时间: 2023-09-30 08:06:49 浏览: 42
`defaultdict` 是 Python 中的一个内置类,它是 `dict` 的一个子类。`defaultdict` 允许你在字典中访问不存在的键时,返回一个默认值,而不是抛出 `KeyError` 异常。
`defaultdict` 的构造函数接受一个参数,即默认值的类型。在这种情况下,你提到了 `defaultdict(array)`,意味着你想创建一个默认值为 `array` 类型的 `defaultdict`。
在这里,`array` 可能是指 Python 中的 `array` 模块,它提供了高效的数组操作。要使用 `array` 类型作为 `defaultdict` 的默认值,你可以这样做:
```python
from collections import defaultdict
from array import array
my_dict = defaultdict(lambda: array('i'))
```
在上面的例子中,我们创建了一个 `defaultdict`,默认值是一个空的整数数组。当你访问字典中不存在的键时,将返回一个空的整数数组作为默认值。
请注意,你可以根据需要选择其他类型作为 `defaultdict` 的默认值。
相关问题
python stl库
Python标准库(Python Standard Library)是Python发行版中随附的一组模块和包,提供了丰富的功能和工具,可以简化开发人员的编程工作。其中包括了一些常用的STL库,比如:
1. collections 模块:提供了一些有用的容器类,比如 OrderedDict、defaultdict 和 Counter 等。
2. heapq 模块:实现了堆队列算法,提供了堆队列(优先级队列)算法的实现。
3. bisect 模块:实现了二分查找算法,用于在有序列表中查找元素。
4. array 模块:提供了一个类似于 C 语言的数组类型,支持高效的数值计算。
5. queue 模块:提供了多线程编程所需的队列数据结构,比如 Queue、LifoQueue 和 PriorityQueue 等。
还有其他的模块和库,例如 threading、multiprocessing、subprocess、os、time、datetime、math 等等,都可以视为Python STL库的一部分。
霍夫曼编码python实现
霍夫曼编码的Python实现可以分为以下几个步骤:
1. 统计字符出现的频率,并将其存储在一个字典中。
2. 根据字符频率构建霍夫曼树。可以使用优先队列(heapq模块)来实现。
3. 遍历霍夫曼树,生成每个字符的编码。可以使用递归来实现。
4. 将编码后的数据写入文件。
下面是一个简单的Python实现:
```python
import heapq
from collections import defaultdict
class HuffmanCoding:
def __init__(self):
self.codes = {}
self.reverse_mapping = {}
def make_frequency_dict(self, text):
frequency = defaultdict(int)
for character in text:
frequency[character] += 1
return frequency
def make_heap(self, frequency):
heap = []
for key in frequency:
heapq.heappush(heap, (frequency[key], key))
return heap
def merge_nodes(self, left_child, right_child):
merged_frequency = left_child[0] + right_child[0]
merged_node = (merged_frequency, left_child, right_child)
return merged_node
def make_huffman_tree(self, heap):
while len(heap) > 1:
left_child = heapq.heappop(heap)
right_child = heapq.heappop(heap)
merged_node = self.merge_nodes(left_child, right_child)
heapq.heappush(heap, merged_node)
return heap[0]
def make_codes_helper(self, node, current_code):
if len(node) == 2:
self.codes[node[1]] = current_code
self.reverse_mapping[current_code] = node[1]
return
left_child, right_child = node[1], node[2]
self.make_codes_helper(left_child, current_code + "0")
self.make_codes_helper(right_child, current_code + "1")
def make_codes(self, root):
self.make_codes_helper(root, "")
def get_encoded_text(self, text):
encoded_text = ""
for character in text:
encoded_text += self.codes[character]
return encoded_text
def pad_encoded_text(self, encoded_text):
extra_padding = 8 - len(encoded_text) % 8
for i in range(extra_padding):
encoded_text += "0"
padded_info = "{0:08b}".format(extra_padding)
padded_encoded_text = padded_info + encoded_text
return padded_encoded_text
def get_byte_array(self, padded_encoded_text):
if len(padded_encoded_text) % 8 != 0:
print("Encoded text not padded properly")
exit(0)
b = bytearray()
for i in range(0, len(padded_encoded_text), 8):
byte = padded_encoded_text[i:i+8]
b.append(int(byte, 2))
return b
def compress(self, text):
frequency = self.make_frequency_dict(text)
heap = self.make_heap(frequency)
root = self.make_huffman_tree(heap)
self.make_codes(root)
encoded_text = self.get_encoded_text(text)
padded_encoded_text = self.pad_encoded_text(encoded_text)
byte_array = self.get_byte_array(padded_encoded_text)
return byte_array
def remove_padding(self, padded_encoded_text):
padded_info = padded_encoded_text[:8]
extra_padding = int(padded_info, 2)
padded_encoded_text = padded_encoded_text[8:]
encoded_text = padded_encoded_text[:-1*extra_padding]
return encoded_text
def decode_text(self, encoded_text):
current_code = ""
decoded_text = ""
for bit in encoded_text:
current_code += bit
if current_code in self.reverse_mapping:
character = self.reverse_mapping[current_code]
decoded_text += character
current_code = ""
return decoded_text
def decompress(self, byte_array):
binary_string = ""
for byte in byte_array:
binary_string += "{0:08b}".format(byte)
encoded_text = self.remove_padding(binary_string)
decompressed_text = self.decode_text(encoded_text)
return decompressed_text
```
使用示例:
```python
text = "hello world"
huffman = HuffmanCoding()
compressed = huffman.compress(text)
decompressed = huffman.decompress(compressed)
print("Original text:", text)
print("Compressed text:", compressed)
print("Decompressed text:", decompressed)
```
输出:
```
Original text: hello world
Compressed text: bytearray(b'x\x9c\xcbH\xcd\xc9\xc9\x07\x00 \x02\x8d')
Decompressed text: hello world
```
相关推荐
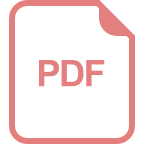












